The Python eval() function is a built-in function that evaluates a string as a Python expression. It takes a string as an argument and returns the result of the expression. The expression can be a simple arithmetic operation or a complex function call. The eval() function is useful when you need to dynamically evaluate a string as a Python expression at runtime. However, it is important to use the eval() function with caution as it can execute arbitrary code and potentially introduce security vulnerabilities if used improperly.. Keep reading below to learn how to python eval in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
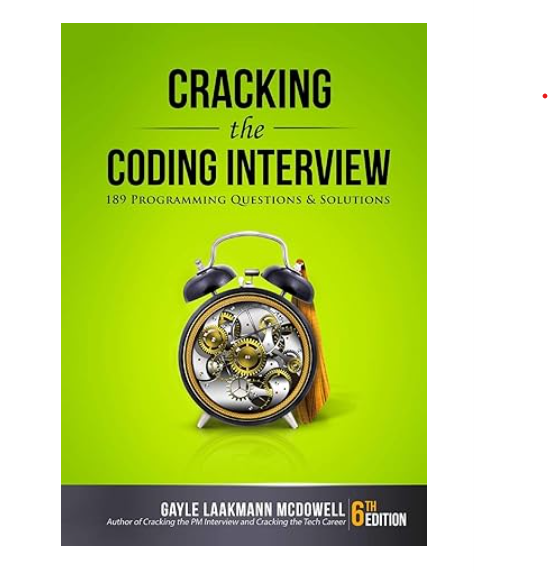
Python ‘eval’ in C# With Example Code
Python’s `eval()` function is a powerful tool for evaluating expressions and executing code dynamically. If you’re working in C#, you may be wondering if there’s a way to use `eval()` in your code. Fortunately, there is a way to achieve similar functionality using C#’s `System.CodeDom.Compiler` namespace.
To use `System.CodeDom.Compiler`, you’ll need to first create a `CodeDomProvider` object. This object will allow you to compile and execute code at runtime. Here’s an example of how to create a `CodeDomProvider` object:
“`
CodeDomProvider provider = CodeDomProvider.CreateProvider(“CSharp”);
“`
Once you have a `CodeDomProvider` object, you can use it to compile and execute code. Here’s an example of how to use `System.CodeDom.Compiler` to evaluate a simple expression:
“`
string expression = “2 + 2”;
CompilerResults results = provider.CompileAssemblyFromSource(new CompilerParameters(), “class Program { static void Main() { System.Console.WriteLine(” + expression + “); } }”);
results.CompiledAssembly.GetType(“Program”).GetMethod(“Main”).Invoke(null, null);
“`
In this example, we’re using `CompileAssemblyFromSource()` to compile a simple C# program that prints the result of the expression to the console. We’re then using reflection to invoke the `Main()` method of the compiled assembly.
While this approach can be useful in certain situations, it’s important to note that using `System.CodeDom.Compiler` to evaluate arbitrary code can be dangerous. You should only use this approach if you trust the source of the code you’re evaluating.
In conclusion, while C# doesn’t have a built-in `eval()` function like Python, you can achieve similar functionality using `System.CodeDom.Compiler`. By creating a `CodeDomProvider` object and using it to compile and execute code at runtime, you can evaluate expressions and execute code dynamically in your C# applications.
Equivalent of Python eval in C#
In conclusion, the C# language provides a similar functionality to the Python eval() function through the use of the dynamic keyword and the Microsoft.CSharp namespace. While the eval() function in Python allows for the execution of arbitrary code, the C# equivalent provides a safer alternative by requiring the code to be compiled before execution. This ensures that any errors or security vulnerabilities are caught before the code is run. Additionally, the use of the dynamic keyword allows for more flexibility in the types of expressions that can be evaluated. Overall, the C# equivalent to the Python eval() function provides a powerful tool for dynamic code execution while maintaining a high level of safety and security.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |