The Python eval() function is a built-in function that evaluates a string as a Python expression. It takes a string as an argument and returns the result of the expression. The expression can be a simple arithmetic operation or a complex function call. The eval() function is useful when you need to dynamically evaluate a string as a Python expression at runtime. However, it is important to use the eval() function with caution as it can execute arbitrary code and potentially introduce security vulnerabilities if used improperly. Keep reading below to learn how to python eval in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
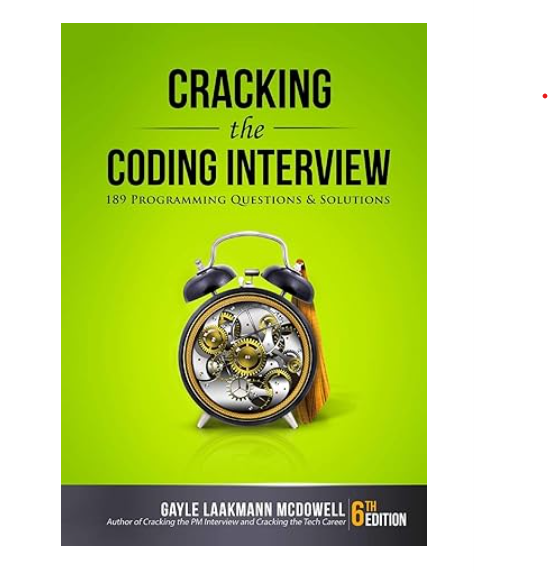
Python ‘eval’ in Kotlin With Example Code
Python’s `eval()` function is a powerful tool for evaluating expressions dynamically. Kotlin, being a language that runs on the JVM, can also make use of this function. In this blog post, we will explore how to use Python’s `eval()` function in Kotlin.
To use `eval()` in Kotlin, we first need to import the `javax.script` package. This package provides a scripting engine that can evaluate expressions in various languages, including Python. Here’s an example of how to use `eval()` in Kotlin:
import javax.script.ScriptEngineManager
fun main() {
val engine = ScriptEngineManager().getEngineByName("python")
val result = engine.eval("2 + 2")
println(result)
}
In this example, we create a new `ScriptEngineManager` and get an instance of the Python engine by name. We then call `eval()` on the engine and pass in the expression we want to evaluate as a string. The result of the evaluation is returned as an object, which we can then print to the console.
It’s important to note that using `eval()` can be dangerous if you’re evaluating user input or untrusted code. It’s recommended to use it only in controlled environments where you can ensure the safety of the input.
In conclusion, Kotlin’s ability to use Python’s `eval()` function provides a powerful tool for evaluating expressions dynamically. By importing the `javax.script` package and using the Python engine, we can easily evaluate Python expressions in Kotlin.
Equivalent of Python eval in Kotlin
In conclusion, the Kotlin programming language provides a powerful and flexible alternative to Python’s eval function with its own built-in function called “evaluate.” This function allows developers to dynamically evaluate expressions and statements at runtime, just like eval in Python. However, unlike eval, evaluate is designed to be safer and more secure, with built-in safeguards to prevent code injection attacks. Additionally, Kotlin’s evaluate function is more type-safe, allowing developers to specify the expected return type of the evaluated expression. Overall, Kotlin’s evaluate function is a valuable tool for developers who need to dynamically evaluate code at runtime, while also ensuring the safety and security of their applications.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |