The Python filter() function is a built-in function that takes two arguments: a function and an iterable. It returns an iterator that contains only the elements from the iterable for which the function returns True. The function argument can be a lambda function or a named function. The filter() function is commonly used to filter out unwanted elements from a list or other iterable based on a certain condition. It is a powerful tool for data manipulation and can be used in a variety of applications.. Keep reading below to learn how to python filter in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
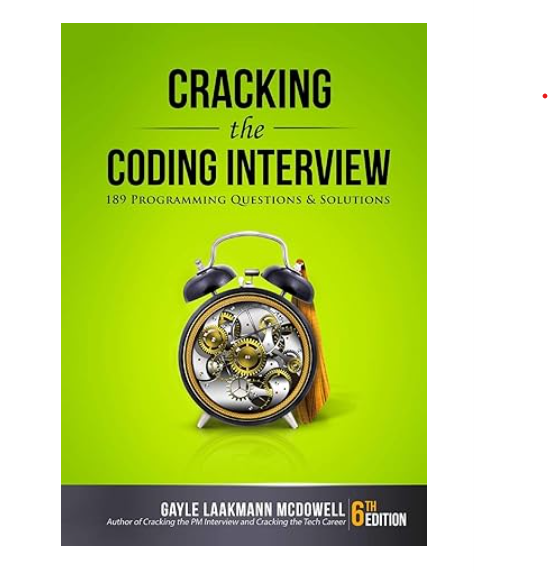
Python ‘filter’ in C# With Example Code
Python is a popular programming language that is widely used for data analysis and machine learning. However, if you are a C# developer, you may wonder how to implement Python’s filter function in C#. In this blog post, we will explore how to achieve this.
First, let’s understand what the filter function does in Python. The filter function takes two arguments: a function and an iterable. It returns an iterator that contains only the elements from the iterable for which the function returns True. For example, the following code filters out all the even numbers from a list:
“`python
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4, 6, 8, 10]
“`
Now, let’s see how we can implement the filter function in C#. C# has a similar function called Where, which is part of the LINQ library. The Where function takes a predicate (a function that returns a Boolean value) and returns an IEnumerable that contains only the elements from the original collection for which the predicate returns true. Here’s an example:
“`csharp
int[] numbers = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10 };
var evenNumbers = numbers.Where(x => x % 2 == 0);
foreach (var number in evenNumbers)
{
Console.WriteLine(number);
}
// Output: 2 4 6 8 10
“`
As you can see, the syntax is slightly different from Python, but the functionality is the same. We use a lambda expression to define the predicate, which checks if the number is even.
In conclusion, while Python’s filter function and C#’s Where function have slightly different syntax, they both provide the same functionality of filtering elements from a collection based on a predicate. By understanding the similarities and differences between these two functions, you can easily implement Python’s filter function in C#.
Equivalent of Python filter in C#
In conclusion, the equivalent of the Python filter function in C# is the LINQ Where method. Both functions allow for filtering of data based on a specified condition, but the syntax and implementation differ slightly between the two languages. While Python’s filter function is a built-in function that can be used with any iterable object, C#’s Where method is part of the LINQ library and can be used with any collection that implements the IEnumerable interface. Despite these differences, both functions are powerful tools for data manipulation and can greatly simplify the process of filtering data in a program. Whether you are working with Python or C#, understanding the filter function and its equivalent in your language of choice can help you write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |