The Python filter() function is a built-in function that takes two arguments: a function and an iterable. It returns an iterator that contains only the elements from the iterable for which the function returns True. The function argument can be a lambda function or a named function. The filter() function is commonly used to filter out unwanted elements from a list or other iterable based on a certain condition. It is a powerful tool for data manipulation and can be used in a variety of applications.. Keep reading below to learn how to python filter in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
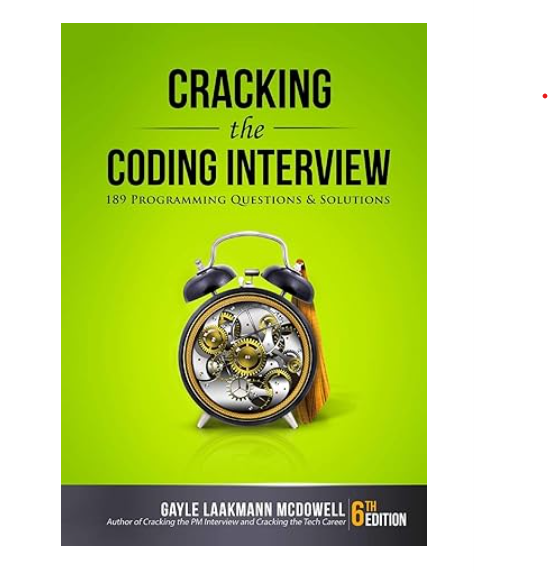
Python ‘filter’ in C++ With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. One of the most useful features of Python is its built-in filter function, which allows you to easily filter a list based on a given condition. If you are a C++ programmer, you may be wondering if there is a similar function in C++. The good news is that there is! In this blog post, we will show you how to implement a Python-style filter function in C++.
To implement a filter function in C++, we will use the standard library function std::remove_if. This function takes two iterators and a predicate function as arguments. The iterators define the range of elements to be filtered, and the predicate function defines the condition that each element must satisfy in order to be included in the filtered range.
Here is an example of how to use std::remove_if to filter a vector of integers:
“`cpp
#include
#include
#include
bool is_even(int n) {
return n % 2 == 0;
}
int main() {
std::vector
auto new_end = std::remove_if(nums.begin(), nums.end(), is_even);
nums.erase(new_end, nums.end());
for (auto n : nums) {
std::cout << n << " ";
}
return 0;
}
```
In this example, we define a predicate function called is_even that returns true if a given integer is even. We then create a vector of integers called nums and use std::remove_if to filter out all even numbers from the vector. Finally, we use the erase function to remove the filtered elements from the vector and print out the remaining elements.
As you can see, implementing a filter function in C++ is quite simple using the std::remove_if function. By defining a custom predicate function, you can easily filter any range of elements based on any condition you like.
Equivalent of Python filter in C++
In conclusion, the equivalent of the Python filter function in C++ is the std::remove_if function. Both functions allow for the filtering of elements in a container based on a given condition. However, the syntax and usage of these functions differ slightly due to the differences in the programming languages. While the Python filter function takes in a lambda function and an iterable object, the C++ std::remove_if function takes in a predicate function and a range of elements. Despite these differences, both functions serve the same purpose of filtering elements in a container and can be used effectively in their respective programming languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |