The Python format() function is a built-in method that allows you to format strings in a specific way. It takes one or more arguments and returns a formatted string. The format() function uses placeholders, which are enclosed in curly braces, to indicate where the values should be inserted. You can specify the order of the values, use named placeholders, and format numbers and strings in various ways. The format() function is a powerful tool for creating dynamic and readable output in your Python programs. Keep reading below to learn how to python format in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
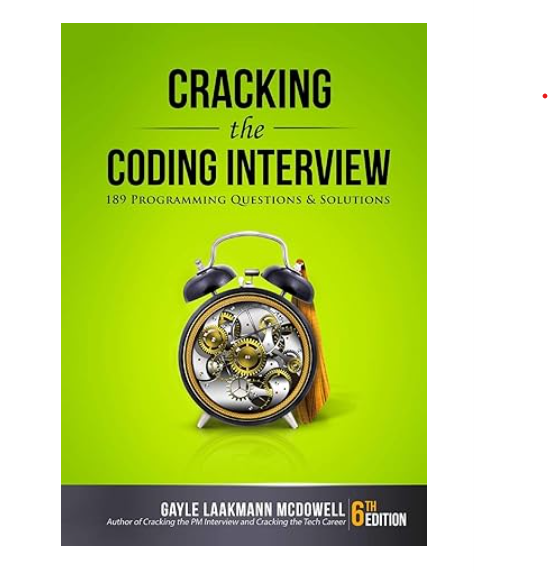
Python ‘format’ in C++ With Example Code
Python is a popular programming language known for its simplicity and ease of use. One of the features that makes Python so easy to use is its string formatting capabilities. Fortunately, C++ also has a similar feature that allows you to format strings in a similar way to Python.To format strings in C++, you can use the `std::format` function, which was introduced in C++20. This function works similarly to Python's `format` method, allowing you to insert values into a string using placeholders.
Here's an example of how to use `std::format`:
#include <format>
#include <iostream>int main() {
std::string name = "John";
int age = 30;
std::string message = std::format("My name is {} and I am {} years old.", name, age);
std::cout << message << std::endl; return 0; }In this example, we're using `std::format` to insert the values of `name` and `age` into the string "My name is {} and I am {} years old." The curly braces {} act as placeholders for the values we want to insert.
When we run this program, we'll see the following output:
My name is John and I am 30 years old.
As you can see, the values of `name` and `age` have been inserted into the string using the placeholders.
In addition to simple placeholders, `std::format` also supports more advanced formatting options, such as specifying the width and precision of numbers, and aligning text. You can learn more about these options in the C++ documentation.
Overall, using `std::format` in C++ is a great way to format strings in a way that's similar to Python's string formatting capabilities. With this feature, you can easily insert values into strings and create dynamic messages and output.
Equivalent of Python format in C++
In conclusion, the equivalent of Python's format function in C++ is the std::format function. This function allows for easy and efficient string formatting in C++, similar to how the format function works in Python. With the std::format function, C++ developers can easily format strings with placeholders and arguments, making their code more readable and maintainable. Additionally, the std::format function is part of the C++20 standard, which means it is widely supported by modern C++ compilers. Overall, the std::format function is a valuable tool for C++ developers who want to improve their string formatting capabilities and streamline their code.
Elevate your software skills
Ergonomic Mouse Custom Keyboard SW Architecture Clean Code