The Python format() function is a built-in method that allows you to format strings in a specific way. It takes one or more arguments and returns a formatted string. The format() function uses placeholders, which are enclosed in curly braces, to indicate where the values should be inserted. You can specify the order of the values, use named placeholders, and format numbers and strings in various ways. The format() function is a powerful tool for creating dynamic and readable output in your Python programs. Keep reading below to learn how to python format in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
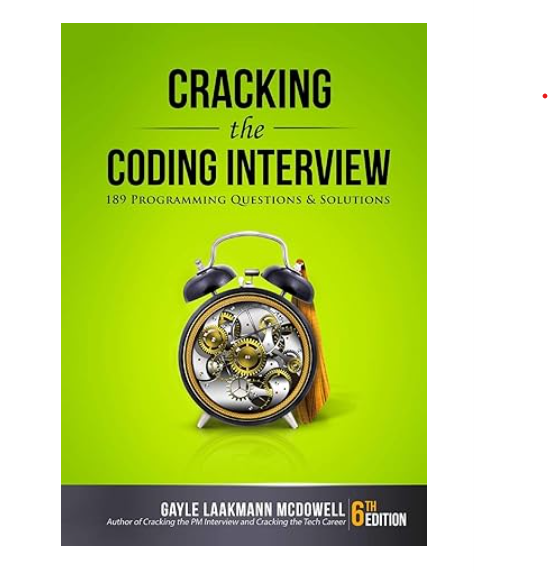
Python ‘format’ in Java With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. One of the features that makes Python so easy to use is its string formatting capabilities. Java, on the other hand, is a more complex language that does not have the same built-in string formatting capabilities as Python. However, there are ways to achieve similar functionality in Java.
One way to format strings in Java is to use the String.format() method. This method works similarly to Python's string formatting syntax, using placeholders and format specifiers to insert values into a string. For example, the following code snippet demonstrates how to use String.format() to format a string with a variable:
String name = "John";
int age = 30;
String message = String.format("My name is %s and I am %d years old.", name, age);
System.out.println(message);
This code will output the following string:
"My name is John and I am 30 years old."
In this example, the %s and %d placeholders are used to insert the values of the name and age variables, respectively. The String.format() method can also be used to format numbers, dates, and other types of data.
Another way to format strings in Java is to use the MessageFormat class. This class provides a more flexible way to format strings, allowing you to specify the order and format of the values being inserted. For example, the following code snippet demonstrates how to use MessageFormat to format a string with two variables:
String name = "John";
int age = 30;
String message = MessageFormat.format("My name is {0} and I am {1,number,#} years old.", name, age);
System.out.println(message);
This code will output the same string as the previous example:
"My name is John and I am 30 years old."
In this example, the {0} and {1,number,#} placeholders are used to insert the values of the name and age variables, respectively. The {1,number,#} placeholder specifies that the second variable should be formatted as a number with no decimal places.
In conclusion, while Java does not have the same built-in string formatting capabilities as Python, there are ways to achieve similar functionality using the String.format() method and the MessageFormat class. These tools can help make your Java code more readable and maintainable, and can save you time and effort when working with strings.
Equivalent of Python format in Java
In conclusion, while Python's `format()` function is a powerful tool for string formatting, Java also offers a similar functionality through its `String.format()` method. Both functions allow for the insertion of variables and values into a string, and offer a range of formatting options to customize the output. While the syntax and usage may differ slightly between the two languages, the end result is the same: a formatted string that can be easily manipulated and displayed. Whether you're working with Python or Java, understanding the capabilities of these string formatting functions can greatly enhance your programming skills and make your code more efficient and readable.
Elevate your software skills
Ergonomic Mouse
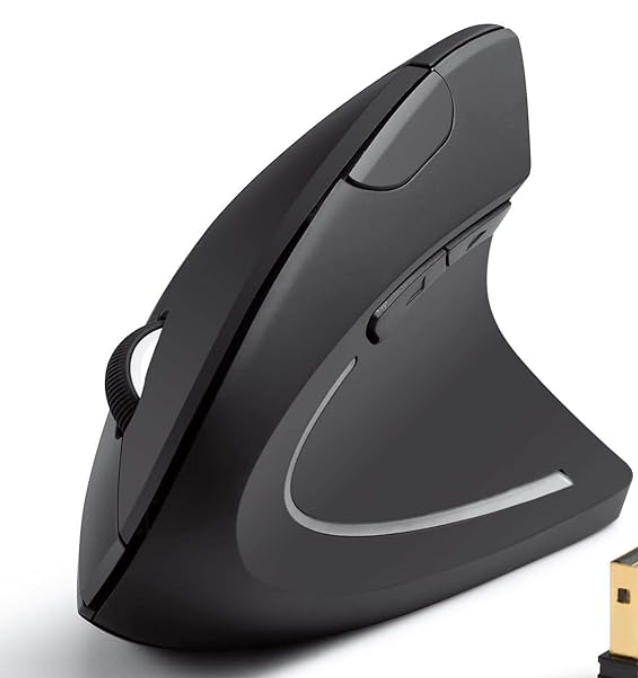
Custom Keyboard
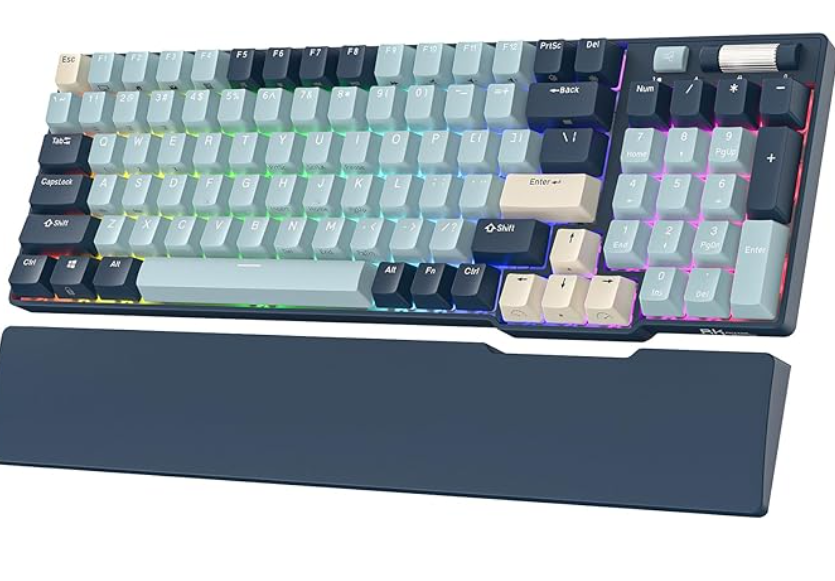
SW Architecture
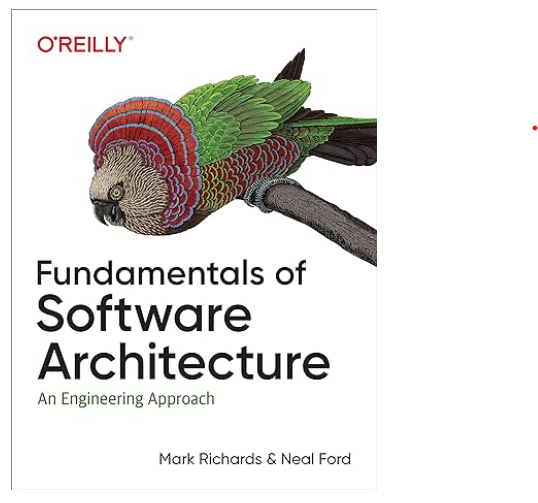
Clean Code
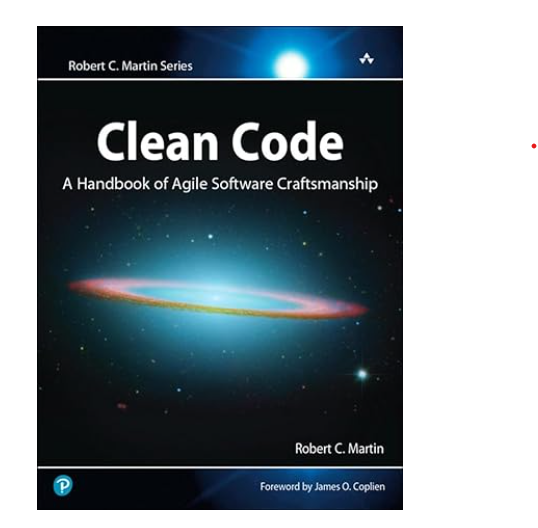