The Python hash function is a built-in function that takes an object as input and returns a unique integer value that represents the object. The hash value is used to quickly compare and identify objects in data structures like dictionaries and sets. The hash function uses a mathematical algorithm to convert the object’s data into a fixed-size integer value. The hash value is deterministic, meaning that it will always return the same value for the same object. However, it is not guaranteed to be unique for different objects, which can lead to collisions. To avoid collisions, objects that are used as keys in dictionaries or elements in sets must be immutable. Keep reading below to learn how to python hash in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
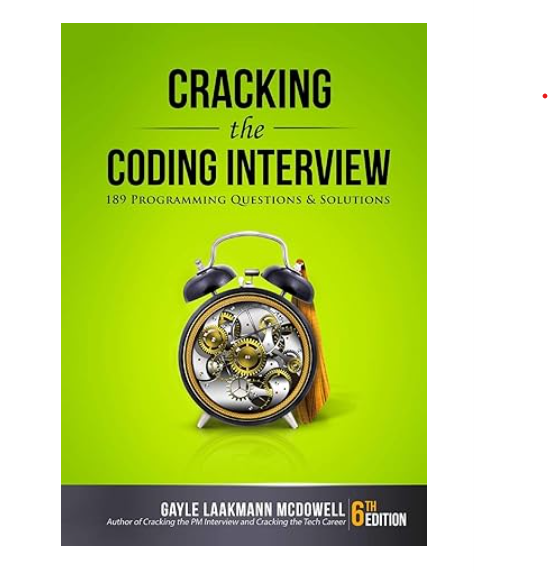
Python ‘hash’ in C++ With Example Code
Python hash in C++ can be a useful tool for developers who want to incorporate Python’s hash function into their C++ code. This can be particularly useful when working with Python libraries or when trying to integrate Python code into a C++ project. In this blog post, we will explore how to use Python’s hash function in C++.
To use Python’s hash function in C++, we first need to include the Python.h header file. This file contains the necessary functions and data structures for working with Python in C++. Here is an example of how to include the Python.h header file:
“`
#include
“`
Once we have included the Python.h header file, we can use the PyObject_Hash() function to hash a Python object. This function takes a PyObject pointer as its argument and returns a hash value as a long integer. Here is an example of how to use the PyObject_Hash() function:
“`
PyObject* obj = Py_BuildValue(“i”, 42);
long hash_value = PyObject_Hash(obj);
“`
In this example, we create a Python integer object with a value of 42 using the Py_BuildValue() function. We then pass this object to the PyObject_Hash() function to get its hash value.
It is important to note that the PyObject_Hash() function can raise a Python exception if the object cannot be hashed. Therefore, it is a good practice to check for errors using the PyErr_Occurred() function after calling PyObject_Hash(). Here is an example of how to check for errors:
“`
PyObject* obj = Py_BuildValue(“i”, 42);
long hash_value = PyObject_Hash(obj);
if (PyErr_Occurred()) {
// Handle error
}
“`
In this example, we check for errors using the PyErr_Occurred() function after calling PyObject_Hash(). If an error occurred, we can handle it appropriately.
In conclusion, using Python’s hash function in C++ can be a powerful tool for developers. By including the Python.h header file and using the PyObject_Hash() function, we can easily hash Python objects in our C++ code. However, it is important to handle errors appropriately to ensure that our code is robust and reliable.
Equivalent of Python hash in C++
In conclusion, the equivalent Python hash function in C++ is a powerful tool for developers who want to implement hashing algorithms in their C++ programs. By using the same hash function as Python, developers can ensure that their code is consistent with Python’s built-in hash function, which can be useful for interoperability between Python and C++ programs. Additionally, the C++ implementation of the Python hash function is highly optimized and can provide fast and efficient hashing for large datasets. Overall, the equivalent Python hash function in C++ is a valuable resource for developers who want to implement hashing algorithms in their C++ programs.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |