The Python id() function returns the unique identifier of an object. This identifier is an integer that is guaranteed to be unique and constant for the lifetime of the object. The id() function can be used to compare two objects to see if they are the same object in memory, as two objects with the same value may have different memory addresses. The id() function can also be used to track the lifetime of an object, as the identifier will change if the object is deleted and then recreated. Overall, the id() function is a useful tool for managing memory and tracking objects in Python. Keep reading below to learn how to python id in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
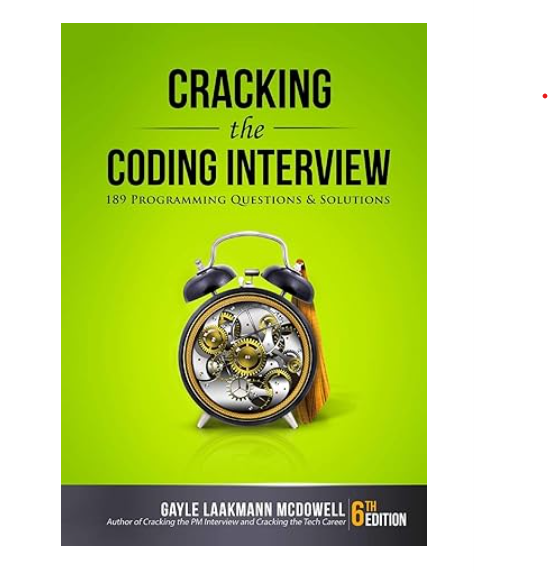
Python ‘id’ in TypeScript With Example Code
Python is a popular programming language that is widely used for various purposes. TypeScript, on the other hand, is a superset of JavaScript that adds optional static typing to the language. In this blog post, we will discuss how to use Python id in TypeScript.
First, let’s understand what Python id does. The id() function in Python returns the identity of an object. This identity is a unique integer that is assigned to the object when it is created. In TypeScript, we can use the typeof operator to get the type of an object. For example:
const obj = { name: "John", age: 30 };
console.log(typeof obj); // output: object
To get the identity of an object in TypeScript, we can use the Object.is() method. This method compares two values and returns true if they are the same value, or false otherwise. For example:
const obj1 = { name: "John", age: 30 };
const obj2 = { name: "John", age: 30 };
console.log(Object.is(obj1, obj2)); // output: false
In the above example, obj1 and obj2 are two different objects with the same values. Therefore, Object.is() returns false.
To get the identity of an object in TypeScript, we can also use the Symbol() function. This function returns a unique symbol value that can be used as an object property. For example:
const id = Symbol();
const obj = { name: "John", age: 30, [id]: 123 };
console.log(obj[id]); // output: 123
In the above example, we create a unique symbol value using the Symbol() function and assign it as a property of the object. We can then access this property using the symbol value.
In conclusion, we can use the Object.is() method or the Symbol() function in TypeScript to get the identity of an object, similar to how we use the id() function in Python.
Equivalent of Python id in TypeScript
In conclusion, TypeScript provides a similar function to Python’s id() function called the “Object.is()” method. This method compares two values and returns a boolean value indicating whether they are the same or not. While it may not provide the exact same functionality as Python’s id() function, it serves a similar purpose in helping developers compare values and determine if they are identical or not. As TypeScript continues to gain popularity among developers, it’s important to understand the various functions and methods available to make coding more efficient and effective. The Object.is() method is just one example of the many useful tools available in TypeScript.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |