The Python input() function is used to take input from the user. It prompts the user to enter a value and waits for the user to input a value from the keyboard. The input() function takes an optional argument, which is the prompt string. This prompt string is displayed to the user before taking input. The input() function returns a string value, which can be stored in a variable for further processing. It is a built-in function in Python and can be used in any Python program. Keep reading below to learn how to python input in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
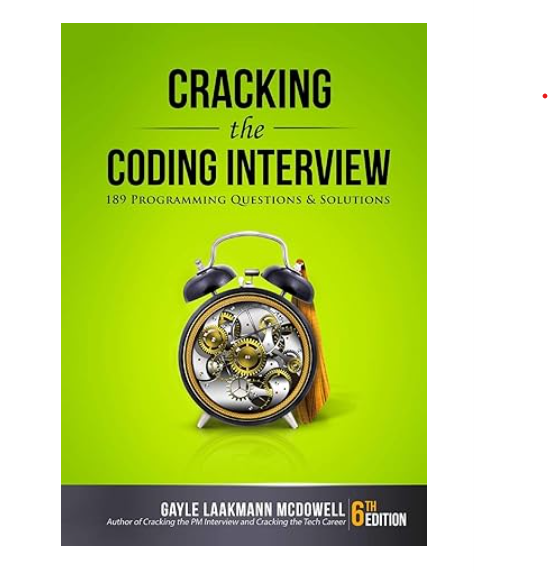
Python ‘input’ in Go With Example Code
Python is a popular programming language used for a variety of applications. However, there may be times when you need to use Go instead. In this blog post, we will discuss how to handle Python input in Go.
First, let’s take a look at how Python handles input. The input() function is used to read a line of text from the user. Here’s an example:
name = input("What is your name? ")
This code prompts the user to enter their name and stores it in the variable “name”.
Now, let’s see how we can achieve the same thing in Go. Go does not have a built-in function for reading input from the user, so we need to use a package called “bufio”. Here’s an example:
package main
import (
"bufio"
"fmt"
"os"
)
func main() {
reader := bufio.NewReader(os.Stdin)
fmt.Print("Enter your name: ")
name, _ := reader.ReadString('\n')
fmt.Println("Hello, " + name)
}
In this code, we import the “bufio”, “fmt”, and “os” packages. We then create a new reader using the “bufio.NewReader()” function and pass in “os.Stdin” as the input source. We then prompt the user to enter their name using “fmt.Print()” and read their input using “reader.ReadString(‘\n’)”. Finally, we print out a greeting using their name.
And that’s it! With the “bufio” package, we can easily handle Python input in Go.
Equivalent of Python input in Go
In conclusion, the equivalent of the Python input() function in Go is the fmt.Scanln() function. Both functions allow the user to input data from the console and store it in a variable. However, there are some differences between the two functions. The input() function in Python automatically converts the user input to a string, while the Scanln() function in Go requires the programmer to specify the data type of the input. Additionally, the input() function in Python allows the user to input multiple values at once, separated by spaces, while the Scanln() function in Go requires the user to input each value on a separate line. Despite these differences, both functions serve the same purpose and are essential tools for console-based programming in their respective languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |