The Python input() function is used to take input from the user. It prompts the user to enter a value and waits for the user to input a value from the keyboard. The input() function takes an optional argument, which is the prompt string. This prompt string is displayed to the user before taking input. The input() function returns a string value, which can be stored in a variable for further processing. It is a built-in function in Python and can be used in any Python program. Keep reading below to learn how to python input in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
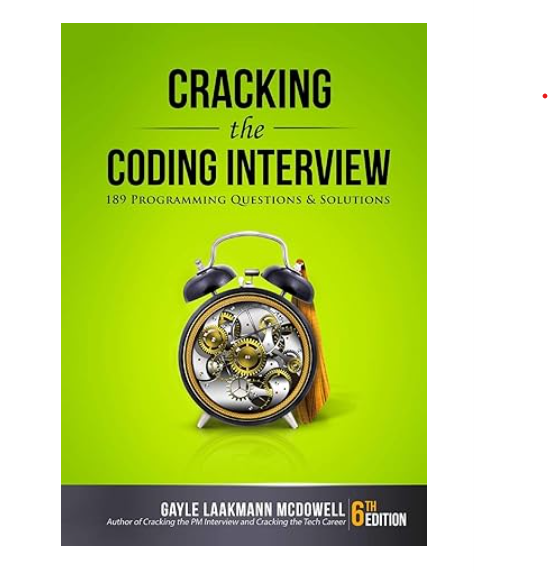
Python ‘input’ in Rust With Example Code
Python is a popular programming language known for its simplicity and ease of use. Rust, on the other hand, is a systems programming language that focuses on performance and safety. While these two languages may seem very different, it is possible to use Python input in Rust.
To use Python input in Rust, you will need to use the `pyo3` crate. This crate provides Rust bindings for the Python interpreter, allowing you to call Python functions and use Python objects from Rust.
To get started, you will need to install the `pyo3` crate by adding it to your `Cargo.toml` file:
[dependencies]
pyo3 = "0.14.2"
Once you have installed the `pyo3` crate, you can use it to call Python functions from Rust. For example, let’s say you have a Python script that prompts the user for their name and then prints a greeting:
name = input("What is your name? ")
print("Hello, " + name + "!")
To call this script from Rust, you can use the `Python::with_gil` function to acquire the Global Interpreter Lock (GIL) and then call the `run` function to execute the script:
use pyo3::prelude::*;
fn main() -> PyResult<()> {
Python::with_gil(|py| {
let globals = py.import("__main__")?.dict();
globals.set_item("name", "Rustacean")?;
py.run("
name = input('What is your name? ')
print('Hello, ' + name + '!')
", None, Some(&globals))?;
Ok(())
})
}
In this example, we are setting the `name` variable to “Rustacean” before executing the script. When the script prompts the user for their name, we can simply enter our name and the script will print a greeting.
Using Python input in Rust can be a powerful tool for building applications that require user input. With the `pyo3` crate, it is easy to call Python functions and use Python objects from Rust, allowing you to take advantage of the strengths of both languages.
Equivalent of Python input in Rust
In conclusion, the Rust programming language provides a similar input function to Python’s input() function, called the std::io::stdin().read_line() method. This method allows users to read input from the standard input stream and store it in a mutable string variable. While the syntax may differ slightly from Python, the functionality is essentially the same. Rust’s input function is a useful tool for developers who want to create interactive command-line applications or programs that require user input. With Rust’s growing popularity and its focus on performance and safety, it’s worth considering using Rust’s input function for your next project.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |