The Python isinstance() function is used to check if an object is an instance of a specified class or a subclass of that class. It takes two arguments: the object to be checked and the class or tuple of classes to check against. The function returns True if the object is an instance of the specified class or a subclass of that class, and False otherwise. This function is commonly used in object-oriented programming to ensure that a variable or parameter is of the expected type before performing operations on it. Keep reading below to learn how to python isinstance in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
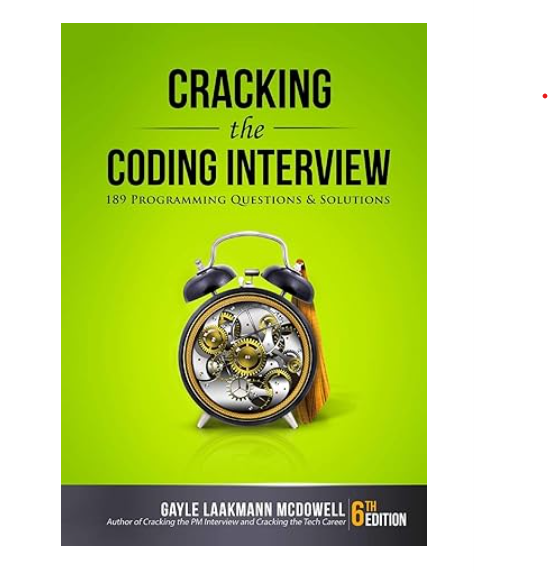
Python ‘isinstance’ in C++ With Example Code
Python’s `isinstance` function is a useful tool for checking the type of an object. It can be particularly helpful when working with dynamic typing in Python. If you’re working in C++, you may be wondering if there’s an equivalent function available. Fortunately, there is! In this post, we’ll explore how to use `isinstance` in C++.
The C++ equivalent of `isinstance` is the `typeid` operator. This operator returns a `type_info` object that contains information about the type of the object passed to it. Here’s an example:
“`
#include
#include
int main() {
int i = 42;
const std::type_info& type = typeid(i);
std::cout << type.name() << std::endl;
return 0;
}
```
In this example, we're using `typeid` to get information about the type of the integer `i`. We're then printing the name of the type to the console using the `name` method of the `type_info` object.
One thing to note is that `typeid` returns a reference to a `const` `type_info` object. This means that you can't modify the object returned by `typeid`.
Another thing to keep in mind is that `typeid` only works with objects that have a runtime type. This means that it won't work with objects that have a static type, such as pointers or references.
Overall, `typeid` is a powerful tool for checking the type of an object in C++. While it may not be as convenient as `isinstance` in Python, it's still a valuable tool to have in your toolbox.
Equivalent of Python isinstance in C++
In conclusion, the equivalent function to Python’s isinstance in C++ is the dynamic_cast operator. This operator allows for dynamic type checking and can be used to determine if an object is of a certain class or a subclass of that class. While it may not be as straightforward as the isinstance function in Python, the dynamic_cast operator provides a powerful tool for C++ developers to ensure type safety and avoid runtime errors. By understanding the differences between these two languages and their respective type checking mechanisms, developers can write more robust and reliable code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |