The Python isinstance() function is used to check if an object is an instance of a specified class or a subclass of that class. It takes two arguments: the object to be checked and the class or tuple of classes to check against. The function returns True if the object is an instance of the specified class or a subclass of that class, and False otherwise. This function is commonly used in object-oriented programming to ensure that a variable or parameter is of the expected type before performing operations on it. Keep reading below to learn how to python isinstance in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
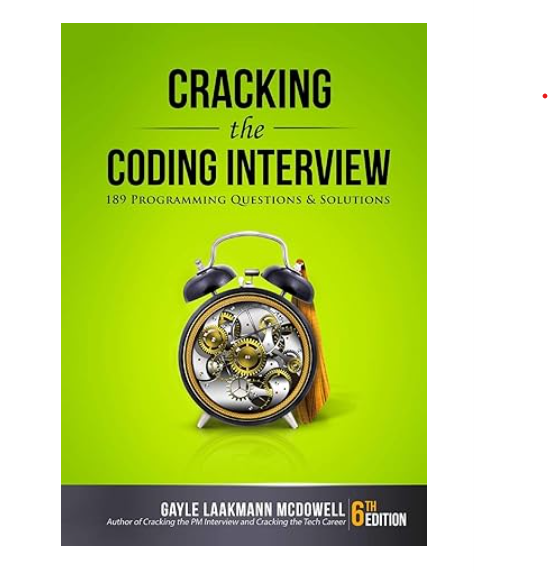
Python ‘isinstance’ in Java With Example Code
Python’s `isinstance` function is a useful tool for checking the type of an object. However, if you are working in Java, you may be wondering how to achieve the same functionality. In this blog post, we will explore how to use `instanceof` in Java to achieve similar results.
In Java, the `instanceof` operator is used to check if an object is an instance of a particular class or interface. The syntax for using `instanceof` is as follows:
“`
object instanceof Class
“`
This will return `true` if the object is an instance of the specified class, or a subclass of the specified class. It will return `false` otherwise.
Here is an example of how to use `instanceof` in Java:
“`
public class Example {
public static void main(String[] args) {
Object obj = “Hello, world!”;
if (obj instanceof String) {
System.out.println(“obj is a String”);
} else {
System.out.println(“obj is not a String”);
}
}
}
“`
In this example, we create an `Object` variable `obj` and assign it the value `”Hello, world!”`. We then use `instanceof` to check if `obj` is a `String`. Since `”Hello, world!”` is a `String`, the output of this program will be `”obj is a String”`.
You can also use `instanceof` to check if an object is an instance of an interface. Here is an example:
“`
public interface MyInterface {
void doSomething();
}
public class Example {
public static void main(String[] args) {
MyInterface obj = new MyClass();
if (obj instanceof MyInterface) {
System.out.println(“obj is an instance of MyInterface”);
} else {
System.out.println(“obj is not an instance of MyInterface”);
}
}
}
public class MyClass implements MyInterface {
public void doSomething() {
System.out.println(“Doing something…”);
}
}
“`
In this example, we define an interface `MyInterface` with a single method `doSomething()`. We then create a class `MyClass` that implements `MyInterface` and overrides the `doSomething()` method. In the `main()` method of the `Example` class, we create an instance of `MyClass` and assign it to an `MyInterface` variable `obj`. We then use `instanceof` to check if `obj` is an instance of `MyInterface`. Since `MyClass` implements `MyInterface`, the output of this program will be `”obj is an instance of MyInterface”`.
In conclusion, while Python’s `isinstance` function may not be available in Java, the `instanceof` operator provides similar functionality for checking the type of an object. By using `instanceof`, you can ensure that your Java code is type-safe and avoid runtime errors.
Equivalent of Python isinstance in Java
In conclusion, while Java does not have an equivalent function to Python’s isinstance(), there are several ways to achieve similar functionality. One approach is to use the getClass() method to check the class of an object, while another is to use the instanceof operator to check if an object is an instance of a particular class or interface. Additionally, Java’s reflection API provides more advanced ways to check the type of an object at runtime. Ultimately, the choice of method will depend on the specific use case and the level of flexibility and precision required. Regardless of the approach taken, it is important to ensure that type checking is done correctly to avoid errors and ensure the proper functioning of the program.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |