The Python issubclass() function is used to check if a given class is a subclass of another class. It takes two arguments, the first being the class to be checked and the second being the class to be checked against. If the first class is a subclass of the second class, the function returns True, otherwise it returns False. This function is useful in object-oriented programming when you need to check if a class inherits from another class or if a particular object belongs to a certain class hierarchy. Keep reading below to learn how to python issubclass in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
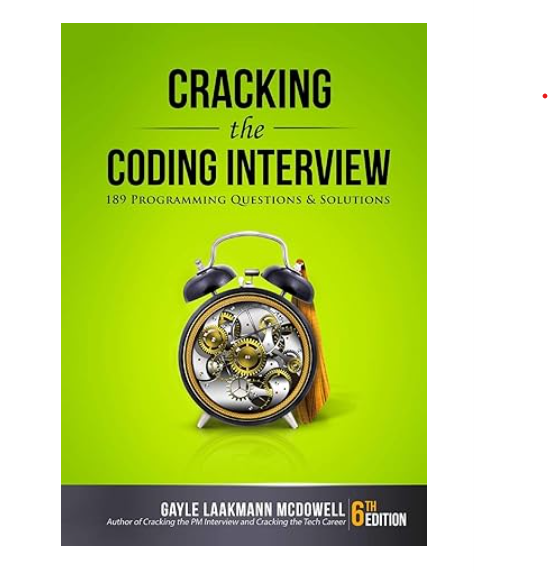
Python ‘issubclass’ in C# With Example Code
Python’s `issubclass` function is a useful tool for checking if a class is a subclass of another class. While C# does not have a built-in function with the same name, we can achieve similar functionality using the `Type.IsSubclassOf` method.
To use `Type.IsSubclassOf`, we first need to get the `Type` objects for the two classes we want to compare. We can do this using the `typeof` operator or the `GetType` method. Once we have the `Type` objects, we can call the `IsSubclassOf` method on the child class’s `Type` object, passing in the parent class’s `Type` object as a parameter.
Here’s an example of how to use `Type.IsSubclassOf` to check if a class is a subclass of another class:
“`
using System;
public class Animal {}
public class Mammal : Animal {}
public class Reptile : Animal {}
public class Program
{
public static void Main()
{
Type mammalType = typeof(Mammal);
Type animalType = typeof(Animal);
Type reptileType = typeof(Reptile);
Console.WriteLine(mammalType.IsSubclassOf(animalType)); // True
Console.WriteLine(reptileType.IsSubclassOf(animalType)); // True
Console.WriteLine(animalType.IsSubclassOf(mammalType)); // False
}
}
“`
In this example, we define three classes: `Animal`, `Mammal`, and `Reptile`. We then use the `typeof` operator to get the `Type` objects for each class. Finally, we call the `IsSubclassOf` method on each `Type` object to check if the class is a subclass of another class.
Note that `Type.IsSubclassOf` only works with classes, not interfaces. If you need to check if a class implements an interface, you can use the `Type.GetInterfaces` method to get an array of the interfaces implemented by the class and then check if the desired interface is in the array.
Equivalent of Python issubclass in C#
In conclusion, the C# programming language provides a similar functionality to Python’s issubclass function through the use of the Type.IsSubclassOf method. This method allows developers to check if a given type is a subclass of another type, providing a useful tool for object-oriented programming. While the syntax and implementation may differ between the two languages, the underlying concept remains the same. By understanding the similarities and differences between these two functions, developers can effectively utilize them in their respective languages to create robust and efficient code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |