The Python issubclass() function is used to check if a given class is a subclass of another class. It takes two arguments, the first being the class to be checked and the second being the class to be checked against. If the first class is a subclass of the second class, the function returns True, otherwise it returns False. This function is useful in object-oriented programming when you need to check if a class inherits from another class or if a particular object belongs to a certain class hierarchy. Keep reading below to learn how to python issubclass in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
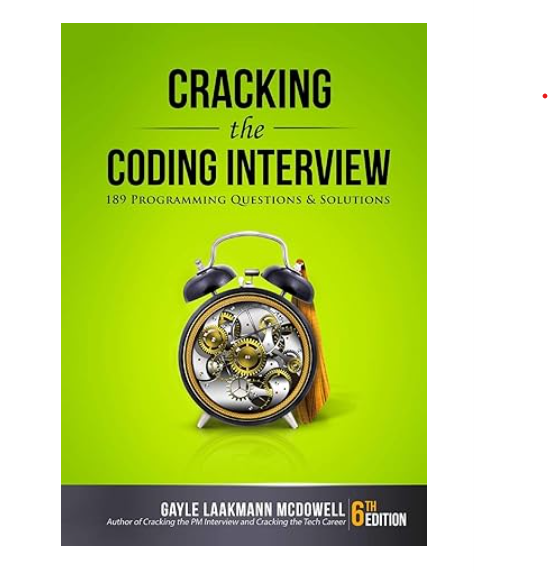
Python ‘issubclass’ in Kotlin With Example Code
Python’s `issubclass` function is a useful tool for checking if a class is a subclass of another class. In Kotlin, there is no direct equivalent to `issubclass`, but there are ways to achieve similar functionality.
One way to check if a class is a subclass of another class in Kotlin is to use the `is` keyword. The `is` keyword can be used to check if an object is an instance of a class or one of its subclasses. For example:
open class Animal
class Dog : Animal()
fun main() {
val dog = Dog()
println(dog is Animal) // true
}
In this example, we define an `Animal` class and a `Dog` class that extends `Animal`. We then create an instance of `Dog` and use the `is` keyword to check if it is an instance of `Animal`. Since `Dog` is a subclass of `Animal`, the output will be `true`.
Another way to achieve similar functionality to `issubclass` in Kotlin is to use reflection. Kotlin’s reflection API allows us to inspect the properties and methods of classes at runtime. We can use reflection to check if a class is a subclass of another class by inspecting its superclass hierarchy. For example:
open class Animal
class Dog : Animal()
fun main() {
val dogClass = Dog::class
val animalClass = Animal::class
println(animalClass.java.isAssignableFrom(dogClass.java)) // true
}
In this example, we use reflection to get the `Class` objects for `Dog` and `Animal`. We then use the `isAssignableFrom` method to check if `Animal` is a superclass of `Dog`. Since `Dog` is a subclass of `Animal`, the output will be `true`.
While Kotlin does not have a direct equivalent to Python’s `issubclass` function, we can use the `is` keyword or reflection to achieve similar functionality.
Equivalent of Python issubclass in Kotlin
In conclusion, the Kotlin programming language provides a powerful and intuitive way to check if a class is a subclass of another class using the `isSubclass` function. This function works similarly to the `issubclass` function in Python, allowing developers to easily check the inheritance relationship between classes. By using this function, Kotlin developers can ensure that their code is efficient, maintainable, and easy to understand. Whether you are a seasoned Kotlin developer or just getting started with the language, the `isSubclass` function is a valuable tool to have in your toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |