The Python issubclass() function is used to check if a given class is a subclass of another class. It takes two arguments, the first being the class to be checked and the second being the class to be checked against. If the first class is a subclass of the second class, the function returns True, otherwise it returns False. This function is useful in object-oriented programming when you need to check if a class inherits from another class or if a particular object belongs to a certain class hierarchy. Keep reading below to learn how to python issubclass in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
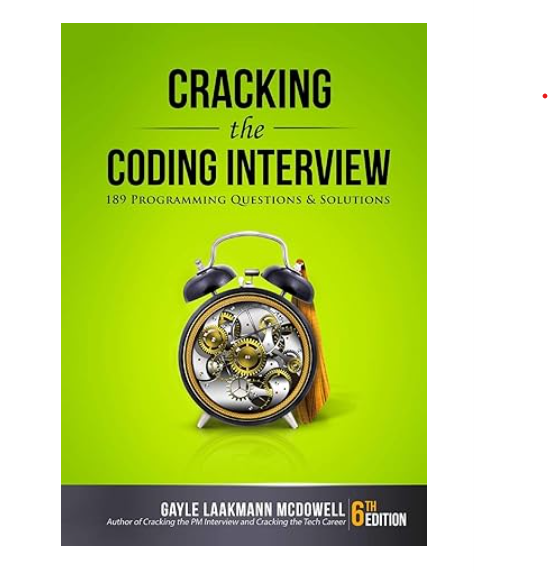
Python ‘issubclass’ in Rust With Example Code
Python’s `issubclass` function is a useful tool for checking if a class is a subclass of another class. Rust, being a different language, does not have this exact function, but there are ways to achieve similar functionality.
One way to check if a Rust struct is a subclass of another struct is to use the `std::any::TypeId` function. This function returns a unique identifier for each type, which can be used to compare if one type is a subclass of another.
Here’s an example of how to use `TypeId` to check if one struct is a subclass of another:
use std::any::TypeId;
struct Animal {}
struct Dog {}
impl Animal {
fn is_subclass_of(&self, other: &Animal) -> bool {
TypeId::of::
}
}
impl Dog {
fn is_subclass_of(&self, other: &Animal) -> bool {
TypeId::of::
}
}
fn main() {
let animal = Animal {};
let dog = Dog {};
assert!(dog.is_subclass_of(&animal));
}
In this example, we define two structs, `Animal` and `Dog`. We then define methods on each struct to check if they are subclasses of `Animal`. The `is_subclass_of` method uses `TypeId` to compare the types of `self` and `other`.
In the `main` function, we create an instance of `Animal` and `Dog`, and then assert that `Dog` is a subclass of `Animal`.
While Rust does not have a built-in `issubclass` function like Python, we can use `TypeId` to achieve similar functionality.
Equivalent of Python issubclass in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to check if a given type is a subclass of another type using the `is_subclass` function. This function is similar to the `issubclass` function in Python and allows developers to easily check the inheritance relationship between two types. With Rust’s strong type system and efficient memory management, the `is_subclass` function can be used to write robust and reliable code. Whether you are a seasoned Rust developer or just getting started, the `is_subclass` function is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |