The Python map() function is a built-in function that applies a given function to each item of an iterable (such as a list, tuple, or set) and returns a new iterable with the results. The syntax for map() is map(function, iterable), where function is the function to apply and iterable is the iterable to apply it to. The function can be a built-in function or a user-defined function. The map() function is useful for performing operations on each item of an iterable without having to write a loop. It can also be used to apply a function to multiple iterables simultaneously. Keep reading below to learn how to python map in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
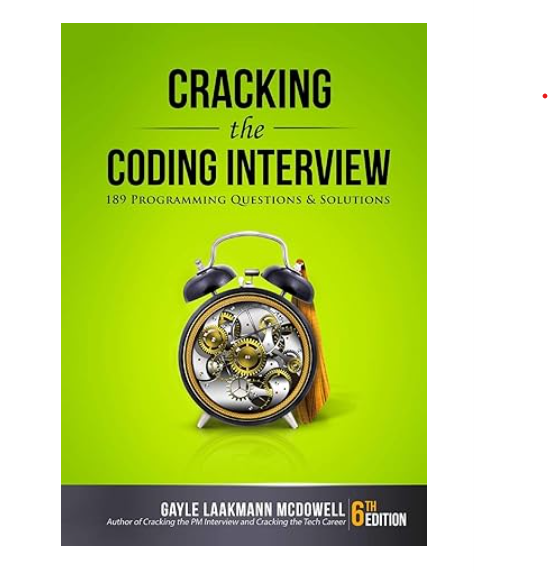
Python ‘map’ in C# With Example Code
Python’s `map()` function is a powerful tool for transforming data. It takes a function and an iterable as arguments, and returns a new iterable with the function applied to each element of the original iterable. This can be a very useful tool for data manipulation and analysis.
If you’re working in C#, you might be wondering if there’s an equivalent to Python’s `map()` function. The good news is that there is! C# has a similar function called `Select()`, which is part of the LINQ library.
Here’s an example of how to use `Select()` in C# to achieve the same result as Python’s `map()` function:
“`
int[] numbers = { 1, 2, 3, 4, 5 };
var squaredNumbers = numbers.Select(x => x * x);
“`
In this example, we have an array of numbers and we want to square each number. We use the `Select()` function to apply the squaring operation to each element of the array, and store the result in a new variable called `squaredNumbers`.
One thing to note is that `Select()` returns an `IEnumerable`, which is similar to Python’s `map()` function returning an iterable. If you want to convert the `IEnumerable` to an array or list, you can use the `ToArray()` or `ToList()` functions, respectively.
Overall, `Select()` is a powerful tool for transforming data in C#, and can be used in a similar way to Python’s `map()` function.
Equivalent of Python map in C#
In conclusion, the equivalent of the Python map function in C# is the LINQ Select method. Both functions allow for the transformation of data in a collection without modifying the original data. The Select method in C# provides a similar level of flexibility and ease of use as the map function in Python. It is a powerful tool for developers who want to manipulate data in a collection and create new collections based on the original data. By using the Select method, developers can write more concise and efficient code, making their programs more readable and maintainable. Overall, the Select method in C# is a valuable addition to any developer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |