The Python map() function is a built-in function that applies a given function to each item of an iterable (such as a list, tuple, or set) and returns a new iterable with the results. The map() function takes two arguments: the first argument is the function to apply, and the second argument is the iterable to apply the function to. The function can be a built-in function or a user-defined function. The map() function returns a map object, which is an iterator that can be converted to a list, tuple, or set. The map() function is useful for applying a function to every element of a list or other iterable without having to write a loop. Keep reading below to learn how to python map in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
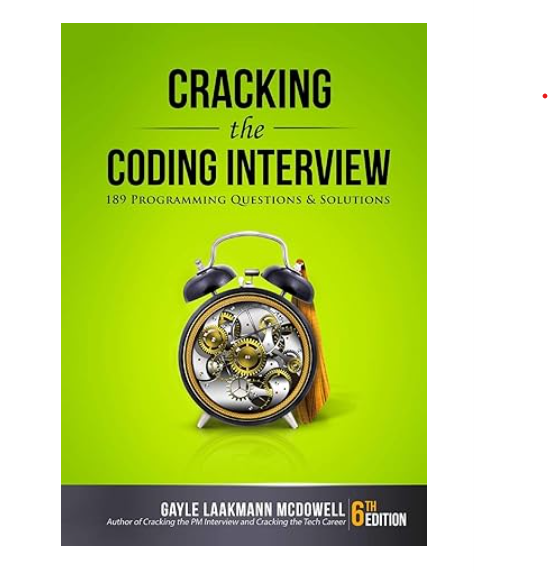
Python ‘map’ in Kotlin With Example Code
Python’s `map()` function is a powerful tool for transforming data. Kotlin, being a language that values concise and expressive code, has its own implementation of `map()` that is just as useful. In this blog post, we’ll explore how to use `map()` in Kotlin.
First, let’s review what `map()` does. In Python, `map()` takes a function and an iterable as arguments, and applies the function to each element of the iterable, returning a new iterable with the transformed values. For example:
def square(x):
return x ** 2
numbers = [1, 2, 3, 4, 5]
squares = map(square, numbers)
print(list(squares)) # [1, 4, 9, 16, 25]
In Kotlin, `map()` is a member function of the `Iterable` interface. It takes a lambda function as an argument, and applies the lambda to each element of the iterable, returning a new iterable with the transformed values. For example:
val numbers = listOf(1, 2, 3, 4, 5)
val squares = numbers.map { it * it }
println(squares) // [1, 4, 9, 16, 25]
Note that in Kotlin, we can use the shorthand `it` instead of defining a separate function for the transformation.
`map()` can also be used with other types of iterables, such as arrays and sequences. Here’s an example using an array:
val numbers = arrayOf(1, 2, 3, 4, 5)
val squares = numbers.map { it * it }
println(squares.toList()) // [1, 4, 9, 16, 25]
And here’s an example using a sequence:
val numbers = generateSequence(1) { it + 1 }
val squares = numbers.take(5).map { it * it }
println(squares.toList()) // [1, 4, 9, 16, 25]
In this example, we’re using `generateSequence()` to create an infinite sequence of numbers, and then using `take()` to limit the sequence to the first five elements.
In conclusion, `map()` is a powerful tool for transforming data in both Python and Kotlin. In Kotlin, it’s a member function of the `Iterable` interface, and takes a lambda function as an argument. With `map()`, we can write concise and expressive code that transforms data with ease.
Equivalent of Python map in Kotlin
In conclusion, the Kotlin programming language provides a powerful and efficient way to perform operations on collections using the `map` function. This function is similar to the `map` function in Python and allows developers to transform each element of a collection into a new element based on a given function. The `map` function in Kotlin is easy to use and provides a concise syntax that makes it a popular choice among developers. With its ability to simplify code and improve performance, the `map` function in Kotlin is a valuable tool for any developer working with collections. Whether you are a beginner or an experienced developer, the `map` function in Kotlin is definitely worth exploring.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |