The Python map() function is a built-in function that applies a given function to each item of an iterable (such as a list, tuple, or set) and returns a new iterable with the results. The map() function takes two arguments: the first argument is the function to apply, and the second argument is the iterable to apply the function to. The function can be a built-in function or a user-defined function. The map() function returns a map object, which is an iterator that can be converted to a list, tuple, or set. The map() function is useful for applying a function to every element of a list or other iterable without having to write a loop. Keep reading below to learn how to python map in PHP.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
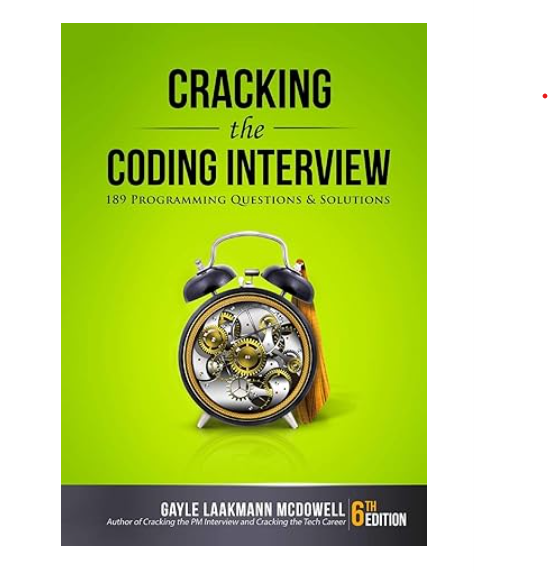
Python ‘map’ in PHP With Example Code
Python’s `map()` function is a powerful tool for manipulating lists and other iterable objects. Fortunately, PHP has a similar function that works in much the same way. In this post, we’ll take a look at how to use the `array_map()` function in PHP to achieve the same results as Python’s `map()`.
The `array_map()` function in PHP takes two arguments: a callback function and an array. The callback function is applied to each element in the array, and the resulting values are returned in a new array. Here’s an example:
function square($n) {
return $n * $n;
}
$numbers = [1, 2, 3, 4, 5];
$squares = array_map("square", $numbers);
print_r($squares);
In this example, we define a function called `square()` that takes a number and returns its square. We then create an array of numbers and use `array_map()` to apply the `square()` function to each element in the array. The resulting array contains the squares of the original numbers.
One thing to note is that the callback function can also be an anonymous function. Here’s an example:
$numbers = [1, 2, 3, 4, 5];
$squares = array_map(function($n) {
return $n * $n;
}, $numbers);
print_r($squares);
In this example, we define an anonymous function that takes a number and returns its square. We then use `array_map()` to apply the anonymous function to each element in the array.
In conclusion, the `array_map()` function in PHP is a powerful tool for manipulating arrays and other iterable objects. By using a callback function, we can achieve the same results as Python’s `map()` function.
Equivalent of Python map in PHP
In conclusion, the PHP language provides a similar function to Python’s map function, which is the array_map() function. This function allows developers to apply a callback function to each element of an array, just like the map function in Python. The array_map() function is a powerful tool that can help developers to manipulate arrays in PHP with ease. It is a great way to save time and effort when working with arrays in PHP. With the array_map() function, developers can easily transform, filter, or modify arrays in PHP, just like they would with the map function in Python. Overall, the array_map() function is a valuable tool for PHP developers who want to work with arrays efficiently and effectively.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |