The Python map() function is a built-in function that applies a given function to each item of an iterable (such as a list, tuple, or set) and returns a new iterable with the results. The map() function takes two arguments: the first argument is the function to apply, and the second argument is the iterable to apply the function to. The function can be a built-in function or a user-defined function. The map() function returns a map object, which is an iterator that can be converted to a list, tuple, or set. The map() function is useful for applying a function to every element of a list or other iterable without having to write a loop. Keep reading below to learn how to python map in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
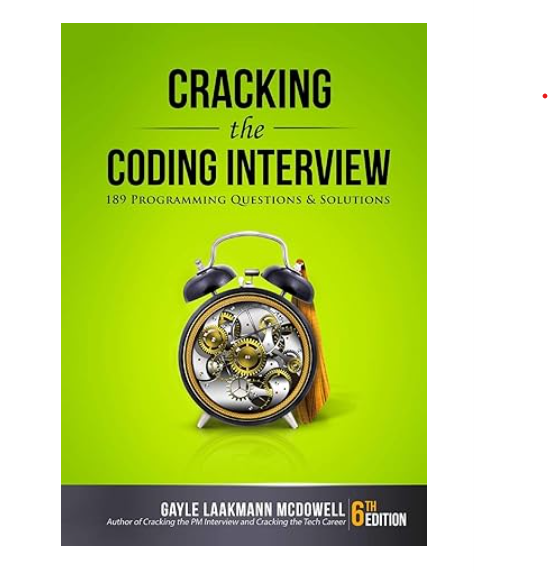
Python ‘map’ in TypeScript With Example Code
Python’s `map()` function is a powerful tool for transforming data. TypeScript, being a superset of JavaScript, also has a `map()` function that can be used in a similar way. In this blog post, we will explore how to use the `map()` function in TypeScript.
The `map()` function in TypeScript works in the same way as the `map()` function in JavaScript. It takes an array and a callback function as arguments. The callback function is called for each element in the array and returns a new value. The `map()` function then returns a new array with the transformed values.
Here is an example of using the `map()` function in TypeScript:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((num) => num * 2);
console.log(doubledNumbers); // Output: [2, 4, 6, 8, 10]
In this example, we have an array of numbers and we want to double each number. We pass a callback function to the `map()` function that takes a number as an argument and returns the number multiplied by 2. The `map()` function then returns a new array with the doubled values.
The `map()` function can also be used with objects. Here is an example:
const users = [
{ name: 'John', age: 25 },
{ name: 'Jane', age: 30 },
{ name: 'Bob', age: 35 },
];
const userNames = users.map((user) => user.name);
console.log(userNames); // Output: ['John', 'Jane', 'Bob']
In this example, we have an array of user objects and we want to extract the names of each user. We pass a callback function to the `map()` function that takes a user object as an argument and returns the name property of the object. The `map()` function then returns a new array with the names of the users.
In conclusion, the `map()` function in TypeScript is a powerful tool for transforming data in arrays and objects. It works in the same way as the `map()` function in JavaScript and can be used in a similar way.
Equivalent of Python map in TypeScript
In conclusion, the TypeScript language provides a powerful and flexible way to work with arrays and collections of data. One of the most useful features of TypeScript is the ability to use the map function, which is similar to the Python map function. With the map function, developers can easily transform and manipulate arrays of data, making it easier to work with complex data structures and perform advanced data processing tasks. Whether you are a seasoned developer or just getting started with TypeScript, the map function is an essential tool that can help you write more efficient and effective code. So, if you’re looking to take your TypeScript skills to the next level, be sure to explore the many benefits of the map function and start using it in your projects today!
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |