The Python max() function is a built-in function that returns the largest item in an iterable or the largest of two or more arguments. It takes one or more arguments and returns the maximum value. If the iterable is empty, it returns a ValueError. The max() function can be used with various data types such as lists, tuples, sets, and dictionaries. It can also be used with custom objects by specifying a key function that returns the value to be compared. The max() function is a useful tool for finding the maximum value in a collection of data. Keep reading below to learn how to python max in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
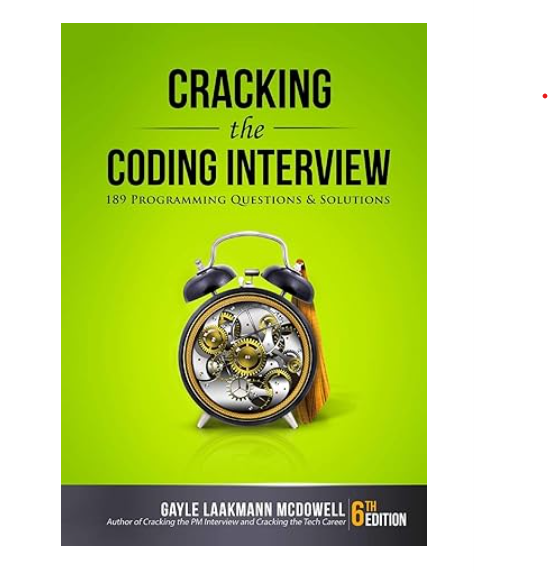
Python ‘max’ in C++ With Example Code
Python’s built-in `max()` function is a convenient way to find the maximum value in a list or iterable. However, if you’re working in C++, you may need to implement this functionality yourself. In this blog post, we’ll explore how to find the maximum value in a C++ array using a similar approach to Python’s `max()` function.
To start, let’s consider a simple array of integers:
“`cpp
int arr[] = { 3, 5, 1, 2, 4 };
“`
Our goal is to find the maximum value in this array. One way to do this is to iterate over the array and keep track of the maximum value we’ve seen so far:
“`cpp
int max_val = arr[0];
for (int i = 1; i < 5; i++) {
if (arr[i] > max_val) {
max_val = arr[i];
}
}
“`
In this code, we initialize `max_val` to the first element of the array. We then iterate over the remaining elements of the array and compare each one to `max_val`. If we find an element that is greater than `max_val`, we update `max_val` to that element.
Once the loop is complete, `max_val` will contain the maximum value in the array. We can print this value to confirm:
“`cpp
std::cout << "The maximum value is: " << max_val << std::endl;
```
And that's it! With this simple approach, we can find the maximum value in any C++ array.
In conclusion, while Python's `max()` function is a convenient way to find the maximum value in a list or iterable, we can achieve similar functionality in C++ by iterating over an array and keeping track of the maximum value we've seen so far.
Equivalent of Python max in C++
In conclusion, the equivalent of the Python max function in C++ is the std::max function. This function is a part of the C++ standard library and can be used to find the maximum value between two or more values. It is a versatile function that can be used with different data types, including integers, floating-point numbers, and even custom data types. The std::max function is a powerful tool for C++ programmers, and it can help simplify code and improve efficiency. By understanding how to use this function, C++ developers can write more efficient and effective code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |