The Python max() function is a built-in function that returns the largest item in an iterable or the largest of two or more arguments. It takes one or more arguments and returns the maximum value. If the iterable is empty, it returns a ValueError. The max() function can be used with various data types such as lists, tuples, sets, and dictionaries. It can also be used with custom objects by specifying a key function that returns the value to be compared. The max() function is a useful tool for finding the maximum value in a collection of data. Keep reading below to learn how to python max in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
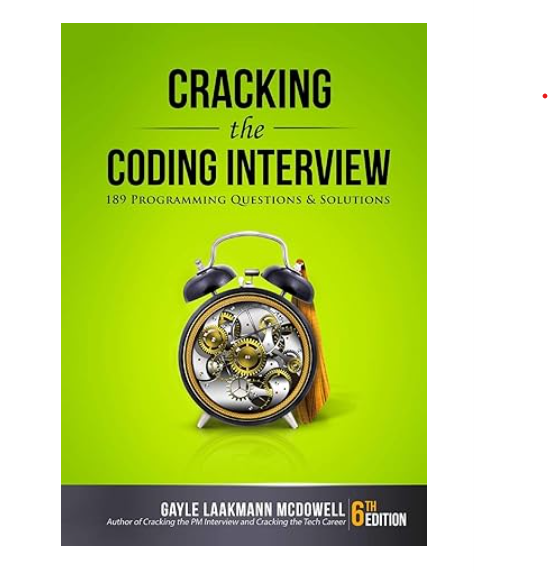
Python ‘max’ in Javascript With Example Code
Python’s built-in `max()` function is a useful tool for finding the highest value in a list or iterable. But what if you’re working in JavaScript and need to find the maximum value in an array? Fortunately, JavaScript has a similar function called `Math.max()`.
To use `Math.max()`, you can pass in one or more arguments representing the values you want to compare. For example, to find the maximum value in an array of numbers, you can use the spread operator (`…`) to pass in each element of the array as a separate argument:
const numbers = [1, 5, 2, 9, 3];
const maxNumber = Math.max(...numbers);
console.log(maxNumber); // Output: 9
If you have an array of objects and want to find the object with the highest value for a specific property, you can use the `Array.reduce()` method to compare each object and return the one with the highest value. For example, let’s say you have an array of objects representing students and their test scores:
const students = [
{ name: 'Alice', score: 85 },
{ name: 'Bob', score: 72 },
{ name: 'Charlie', score: 90 },
{ name: 'Dave', score: 68 }
];
To find the student with the highest score, you can use `Array.reduce()` like this:
const topStudent = students.reduce((prev, current) => {
return (prev.score > current.score) ? prev : current
});
console.log(topStudent.name); // Output: Charlie
In this example, `Array.reduce()` compares each object in the `students` array and returns the one with the highest `score` property. The `prev` and `current` parameters represent the previous and current objects being compared, and the ternary operator (`?`) returns the object with the higher score.
With `Math.max()` and `Array.reduce()`, you can easily find the maximum value in an array or the object with the highest value for a specific property in JavaScript.
Equivalent of Python max in Javascript
In conclusion, the equivalent of the Python max function in JavaScript is the Math.max() method. This method takes in a list of numbers as arguments and returns the highest value among them. It is a simple and efficient way to find the maximum value in an array or a list of numbers in JavaScript. By understanding the similarities and differences between the Python max function and the JavaScript Math.max() method, developers can easily switch between the two languages and achieve the same results. Whether you are working with Python or JavaScript, the max function is an essential tool for finding the highest value in a list or an array.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |