The Python max() function is a built-in function that returns the largest item in an iterable or the largest of two or more arguments. It takes one or more arguments and returns the maximum value. If the iterable is empty, it returns a ValueError. The max() function can be used with various data types such as lists, tuples, sets, and dictionaries. It can also be used with custom objects by specifying a key function that returns the value to be compared. The max() function is a useful tool for finding the maximum value in a collection of data. Keep reading below to learn how to python max in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
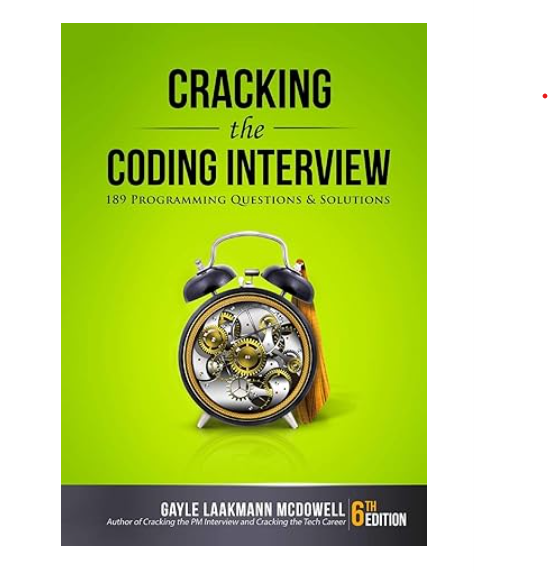
Python ‘max’ in Rust With Example Code
Python’s `max()` function is a useful tool for finding the maximum value in a list or iterable. If you’re working in Rust and need to find the maximum value in a collection, you might be wondering how to achieve the same functionality. Fortunately, Rust provides several ways to find the maximum value in a collection.
One way to find the maximum value in a collection in Rust is to use the `iter()` method to create an iterator over the collection, and then use the `max()` method on the iterator. Here’s an example:
let numbers = vec![1, 2, 3, 4, 5];
let max_number = numbers.iter().max();
In this example, we create a vector of numbers and then create an iterator over the vector using the `iter()` method. We then call the `max()` method on the iterator to find the maximum value in the vector.
Another way to find the maximum value in a collection in Rust is to use the `fold()` method. The `fold()` method allows you to apply a function to each element in a collection and accumulate the results. Here’s an example:
let numbers = vec![1, 2, 3, 4, 5];
let max_number = numbers.iter().fold(0, |a, &b| if a > b { a } else { b });
In this example, we create a vector of numbers and then create an iterator over the vector using the `iter()` method. We then call the `fold()` method on the iterator, passing in an initial value of 0 and a closure that compares each element in the vector to the current maximum value and returns the larger of the two.
These are just two examples of how to find the maximum value in a collection in Rust. Depending on your specific use case, there may be other methods that are more appropriate.
Equivalent of Python max in Rust
In conclusion, the Rust programming language provides a powerful and efficient alternative to Python’s max function. The max function in Rust is implemented using the std::cmp::max function, which takes two arguments and returns the maximum value between them. This function can be used with any data type that implements the PartialOrd trait, making it a versatile tool for comparing and finding the maximum value in a collection of data. Additionally, Rust’s strong type system and memory safety features make it a reliable choice for high-performance applications. Overall, the Rust max function is a valuable addition to any developer’s toolkit, offering a fast and reliable way to find the maximum value in a collection of data.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |