The Python max() function is a built-in function that returns the largest item in an iterable or the largest of two or more arguments. It takes one or more arguments and returns the maximum value. If the iterable is empty, it returns a ValueError. The max() function can be used with various data types such as lists, tuples, sets, and dictionaries. It can also be used with custom objects by specifying a key function that returns the value to be compared. The max() function is a useful tool for finding the maximum value in a collection of data. Keep reading below to learn how to python max in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
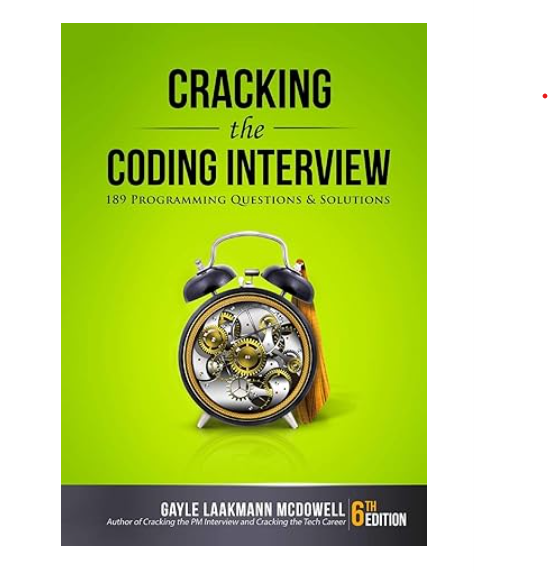
Python ‘max’ in TypeScript With Example Code
Python’s built-in `max()` function is a useful tool for finding the highest value in a list or iterable. However, if you’re working with TypeScript, you may be wondering how to achieve the same functionality. Fortunately, TypeScript provides a way to accomplish this task.
To find the maximum value in an array in TypeScript, you can use the `Math.max()` function. This function takes any number of arguments and returns the highest value. However, it doesn’t work directly with arrays. To use it with an array, you can use the spread operator (`…`) to pass the array’s elements as separate arguments.
Here’s an example of how to use `Math.max()` to find the maximum value in an array:
const numbers = [1, 5, 2, 9, 3];
const maxNumber = Math.max(...numbers);
console.log(maxNumber); // Output: 9
In this example, we define an array of numbers and then use the spread operator to pass each element as a separate argument to `Math.max()`. The function returns the highest value, which we store in the `maxNumber` variable. Finally, we log the result to the console.
It’s worth noting that `Math.max()` returns `-Infinity` if called with no arguments. To avoid this, you can add a check to ensure that the array is not empty before calling the function.
In conclusion, while TypeScript doesn’t have a built-in `max()` function like Python, you can use `Math.max()` with the spread operator to achieve the same functionality.
Equivalent of Python max in TypeScript
In conclusion, TypeScript provides a powerful and efficient way to write type-safe code for JavaScript applications. The equivalent of the Python max function in TypeScript is the Math.max() function, which can be used to find the maximum value in an array of numbers. By using TypeScript’s type annotations and strict type checking, developers can ensure that their code is free from errors and runs smoothly. With its growing popularity and support from major tech companies, TypeScript is quickly becoming a go-to language for building robust and scalable web applications. So, if you’re looking to write clean and reliable code for your next project, consider giving TypeScript a try and make use of its powerful features like the Math.max() function.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |