The Python min() function is a built-in function that returns the smallest item in an iterable or the smallest of two or more arguments. It takes an iterable (such as a list, tuple, or set) or multiple arguments as input and returns the smallest value. If the iterable is empty, it raises a ValueError. The min() function can also take a key argument that specifies a function to be applied to each element before comparison. This allows for more complex comparisons, such as sorting a list of strings by their length. Overall, the min() function is a useful tool for finding the smallest value in a collection of data. Keep reading below to learn how to python min in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
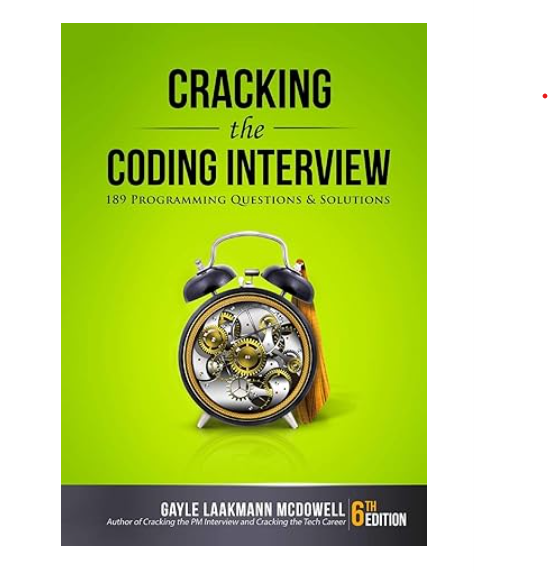
Python ‘min’ in C# With Example Code
Python’s built-in `min` function is a useful tool for finding the smallest value in a list or iterable. However, if you’re working in C#, you may be wondering how to achieve the same functionality. Fortunately, C# provides a similar method for finding the minimum value in a collection: `Enumerable.Min`.
To use `Enumerable.Min`, you’ll need to import the `System.Linq` namespace. Here’s an example of how to find the minimum value in an array of integers:
“`csharp
using System.Linq;
int[] numbers = { 3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5 };
int min = numbers.Min();
Console.WriteLine(min); // Output: 1
“`
In this example, we first import the `System.Linq` namespace. Then, we create an array of integers called `numbers`. We use the `Min` method to find the smallest value in the array, and store it in the `min` variable. Finally, we print the value of `min` to the console.
`Enumerable.Min` can also be used with other types of collections, such as lists or dictionaries. Here’s an example of how to find the minimum value in a list of doubles:
“`csharp
using System.Linq;
using System.Collections.Generic;
List
double minPrice = prices.Min();
Console.WriteLine(minPrice); // Output: 1.99
“`
In this example, we create a list of doubles called `prices`. We use the `Min` method to find the smallest value in the list, and store it in the `minPrice` variable. Finally, we print the value of `minPrice` to the console.
In summary, if you need to find the minimum value in a collection in C#, you can use the `Enumerable.Min` method from the `System.Linq` namespace.
Equivalent of Python min in C#
In conclusion, the equivalent of the Python min function in C# is the Math.Min method. This method takes two arguments and returns the smaller of the two values. It can also be used with an array of values by using the params keyword. While the syntax may be slightly different, the functionality is the same and can be easily implemented in C# code. Whether you are a Python developer transitioning to C# or simply looking to expand your programming knowledge, understanding the equivalent functions in different languages can be a valuable skill.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |