The Python min() function is a built-in function that returns the smallest item in an iterable or the smallest of two or more arguments. It takes an iterable (such as a list, tuple, or set) or multiple arguments as input and returns the smallest value. If the iterable is empty, it raises a ValueError. The min() function can also take a key argument that specifies a function to be applied to each element before comparison. This allows for more complex comparisons, such as sorting a list of strings by their length. Overall, the min() function is a useful tool for finding the smallest value in a collection of data. Keep reading below to learn how to python min in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
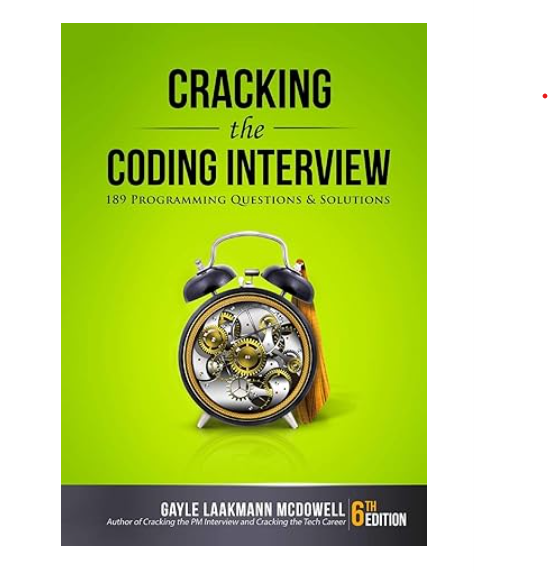
Python ‘min’ in C++ With Example Code
Python’s `min()` function is a built-in function that returns the smallest item in an iterable. In C++, there is no built-in function that does exactly the same thing, but it is easy to implement one.
One way to implement a `min()` function in C++ is to use templates. Templates allow us to write a function that can work with different types of data. Here is an example implementation:
“`cpp In conclusion, the equivalent of the Python min function in C++ is the std::min function. This function is a part of the standard library in C++ and can be used to find the minimum value between two or more values. It is a simple and efficient way to find the minimum value in a given set of values. The std::min function can be used with different data types, including integers, floating-point numbers, and even custom data types. By using this function, C++ programmers can easily find the minimum value in their programs without having to write complex code. Overall, the std::min function is a valuable tool for C++ programmers who need to find the minimum value in their programs.
template
T my_min(T a, T b) {
return (a < b) ? a : b;
}
```
This function takes two arguments of the same type `T` and returns the smaller of the two. The `?:` operator is a shorthand for an `if` statement.
We can use this function to find the minimum of two integers like this:
```cpp
int a = 5;
int b = 3;
int c = my_min(a, b);
```
In this example, `c` will be assigned the value `3`.
We can also use this function to find the minimum of two floating-point numbers:
```cpp
double x = 3.14;
double y = 2.71;
double z = my_min(x, y);
```
In this example, `z` will be assigned the value `2.71`.
In conclusion, while C++ does not have a built-in `min()` function like Python, it is easy to implement one using templates.
Equivalent of Python min in C++
Elevate your software skills
Ergonomic Mouse
Custom Keyboard
SW Architecture
Clean Code