The Python ord() function is a built-in function that returns the Unicode code point of a given character. It takes a single argument, which can be a string of length 1 or a Unicode character. The returned value is an integer representing the Unicode code point of the character. The ord() function is useful when working with Unicode strings and characters, as it allows you to convert characters to their corresponding code points, which can then be used for various operations such as sorting, searching, and encoding. Keep reading below to learn how to python ord in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
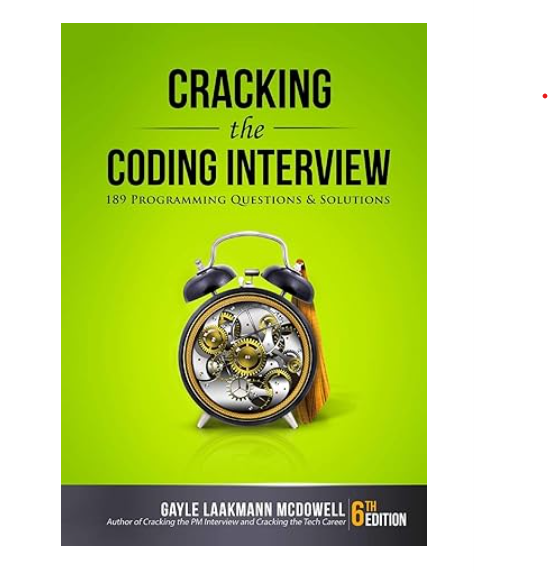
Python ‘ord’ in TypeScript With Example Code
Python’s `ord()` function returns the Unicode code point of a given character. In TypeScript, we can achieve the same functionality using the `charCodeAt()` method of a string.
To get the Unicode code point of a character in TypeScript, we can use the following code:
const char = 'A';
const code = char.charCodeAt(0);
console.log(code); // Output: 65
In the above code, we first define a variable `char` and assign it the value of the character whose Unicode code point we want to find. We then use the `charCodeAt()` method to get the Unicode code point of the character. The `charCodeAt()` method takes an index as an argument, which specifies the position of the character in the string. Since we are only interested in the Unicode code point of a single character, we pass the index `0` to the `charCodeAt()` method.
We can also use a loop to get the Unicode code points of all the characters in a string. Here’s an example:
const str = 'Hello, world!';
for (let i = 0; i < str.length; i++) {
const code = str.charCodeAt(i);
console.log(`Character: ${str[i]}, Code: ${code}`);
}
In the above code, we define a variable `str` and assign it the value of the string whose characters' Unicode code points we want to find. We then use a `for` loop to iterate over each character in the string. Inside the loop, we use the `charCodeAt()` method to get the Unicode code point of the current character. We then log the character and its Unicode code point to the console.
Using the `charCodeAt()` method, we can easily get the Unicode code point of a character or all the characters in a string in TypeScript.
Equivalent of Python ord in TypeScript
In conclusion, the equivalent of the Python `ord()` function in TypeScript is the `charCodeAt()` method. Both functions serve the same purpose of returning the Unicode code point of a given character. However, it is important to note that while `ord()` can accept a string of length 1 or a character, `charCodeAt()` can only accept a character at a specific index in a string. Overall, understanding the equivalent functions in different programming languages can be helpful for developers who work with multiple languages or need to convert code from one language to another. By knowing the equivalent of a function in another language, developers can save time and avoid errors when working on projects that require cross-language compatibility.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |