The Python pow() function is used to calculate the power of a number. It takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent. The pow() function can be used with both integers and floating-point numbers. If the exponent is negative, the pow() function returns the reciprocal of the result. Additionally, the pow() function can take a third argument, which is the modulus. If the modulus is specified, the pow() function returns the result modulo the modulus. Overall, the pow() function is a useful tool for performing power calculations in Python. Keep reading below to learn how to python pow in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
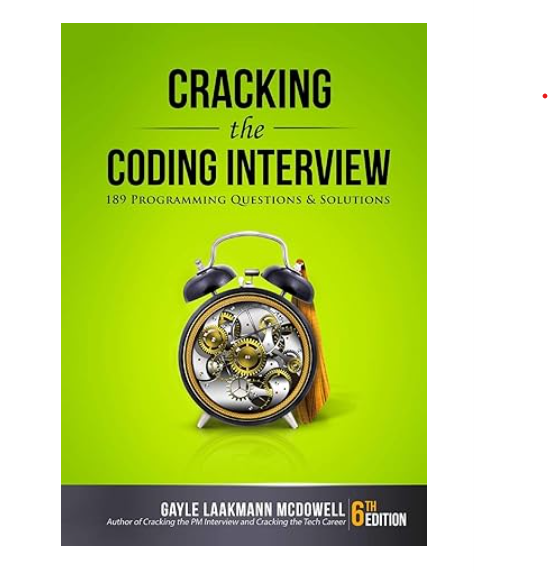
Python ‘pow’ in C# With Example Code
Python’s built-in `pow` function is used to calculate the power of a number. In C#, the equivalent function is `Math.Pow`.
To use `Math.Pow`, you need to provide two arguments: the base number and the exponent. For example, to calculate 2 raised to the power of 3, you would use the following code:
“`csharp
double result = Math.Pow(2, 3);
“`
This would set the variable `result` to 8.0.
It’s important to note that `Math.Pow` returns a `double` value, so you may need to cast the result to a different data type if necessary.
Here’s an example of using `Math.Pow` in a program to calculate the area of a circle:
“`csharp
using System;
class Program
{
static void Main()
{
double radius = 5.0;
double area = Math.PI * Math.Pow(radius, 2);
Console.WriteLine(“The area of a circle with radius {0} is {1}”, radius, area);
}
}
“`
In this example, we’re using `Math.PI` to get the value of pi, and then using `Math.Pow` to calculate the area of the circle.
Overall, `Math.Pow` is a useful function in C# for calculating powers of numbers.
Equivalent of Python pow in C#
In conclusion, the equivalent of the Python pow() function in C# is the Math.Pow() method. This method allows us to raise a number to a specified power and obtain the result. It is a built-in function in the C# language and can be used in various applications, including scientific calculations, financial modeling, and game development. The Math.Pow() method takes two arguments, the base number and the exponent, and returns the result as a double data type. With this knowledge, developers can easily perform power calculations in C# and achieve the desired results.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |