The Python pow() function is used to calculate the power of a number. It takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent. The pow() function can be used with both integers and floating-point numbers. If the exponent is negative, the pow() function returns the reciprocal of the result. Additionally, the pow() function can take a third argument, which is the modulus. If the modulus is specified, the pow() function returns the result modulo the modulus. Overall, the pow() function is a useful tool for performing power calculations in Python. Keep reading below to learn how to python pow in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
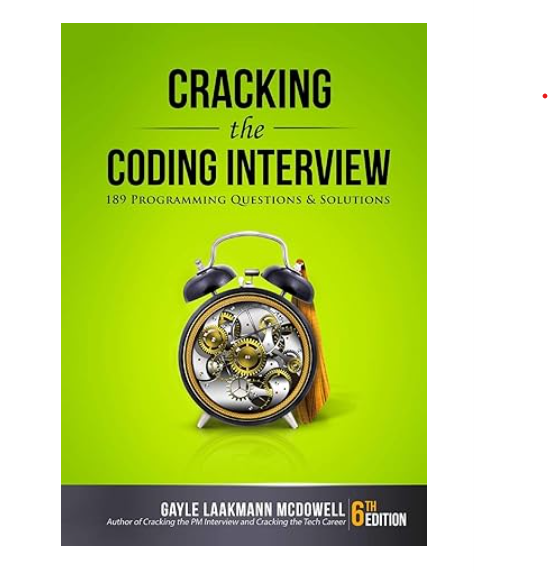
Python ‘pow’ in C++ With Example Code
Python’s built-in `pow()` function is used to calculate the power of a number. In C++, we can achieve the same functionality using the `pow()` function from the `
To use the `pow()` function in C++, we need to include the `
“`cpp
#include
“`
The `pow()` function takes two arguments: the base and the exponent. It returns the result of raising the base to the exponent.
“`cpp
double pow(double base, double exponent);
“`
Here’s an example of using the `pow()` function in C++:
“`cpp
#include
#include
int main() {
double base = 2.0;
double exponent = 3.0;
double result = pow(base, exponent);
std::cout << base << " raised to the power of " << exponent << " is " << result << std::endl;
return 0;
}
```
This will output:
```
2 raised to the power of 3 is 8
```
Note that the `pow()` function returns a `double` value, so we need to use a `double` variable to store the result.
In addition to the `pow()` function, the `
Equivalent of Python pow in C++
In conclusion, the equivalent of the Python pow() function in C++ is the pow() function from the cmath library. This function takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent. It is important to note that the pow() function in C++ returns a double value, so it may not be suitable for all use cases. However, for most applications, the pow() function in C++ is a reliable and efficient way to perform exponentiation. Whether you are working with complex mathematical calculations or simply need to raise a number to a power, the pow() function in C++ is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |