The Python pow() function is used to calculate the power of a number. It takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent. The pow() function can be used with both integers and floating-point numbers. If the exponent is negative, the pow() function returns the reciprocal of the result. Additionally, the pow() function can take a third argument, which is the modulus. If the modulus is specified, the pow() function returns the result modulo the modulus. Overall, the pow() function is a useful tool for performing power calculations in Python. Keep reading below to learn how to python pow in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
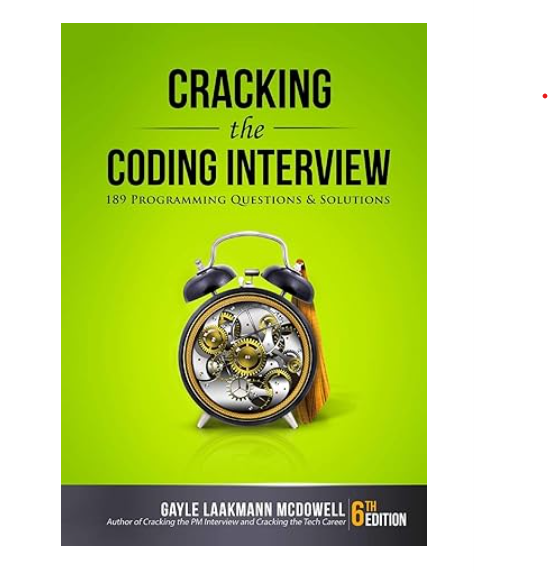
Python ‘pow’ in Rust With Example Code
Python’s built-in `pow` function is used to calculate the power of a number. Rust, being a systems programming language, does not have a built-in `pow` function. However, Rust provides the `pow` method through the `std::ops::Pow` trait.
To use the `pow` method, you need to import the `std::ops::Pow` trait. Here’s an example:
use std::ops::Pow;
fn main() {
let base = 2;
let exponent = 3;
let result = base.pow(exponent);
println!("{} raised to the power of {} is {}", base, exponent, result);
}
In this example, we import the `std::ops::Pow` trait and use the `pow` method to calculate the power of `base` raised to `exponent`. The result is then printed to the console.
You can also use the `powf` method to calculate the power of a floating-point number. Here’s an example:
fn main() {
let base = 2.0;
let exponent = 3.0;
let result = base.powf(exponent);
println!("{} raised to the power of {} is {}", base, exponent, result);
}
In this example, we use the `powf` method to calculate the power of `base` raised to `exponent`. The result is then printed to the console.
In conclusion, Rust provides the `pow` method through the `std::ops::Pow` trait to calculate the power of a number. You can use the `pow` method to calculate the power of an integer and the `powf` method to calculate the power of a floating-point number.
Equivalent of Python pow in Rust
In conclusion, the Rust programming language provides a powerful and efficient way to perform exponentiation with its built-in pow function. This function is equivalent to the pow function in Python and allows developers to easily raise a number to a given power. With Rust’s focus on performance and safety, the pow function is optimized for speed and accuracy, making it a great choice for any project that requires fast and reliable exponentiation. Whether you are a seasoned Rust developer or just getting started, the pow function is a valuable tool to have in your programming arsenal.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |