The Python pow() function is used to calculate the power of a number. It takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent. The pow() function can be used with both integers and floating-point numbers. If the exponent is negative, the pow() function returns the reciprocal of the result. Additionally, the pow() function can take a third argument, which is the modulus. If the modulus is specified, the pow() function returns the result modulo the modulus. Overall, the pow() function is a useful tool for performing power calculations in Python. Keep reading below to learn how to python pow in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
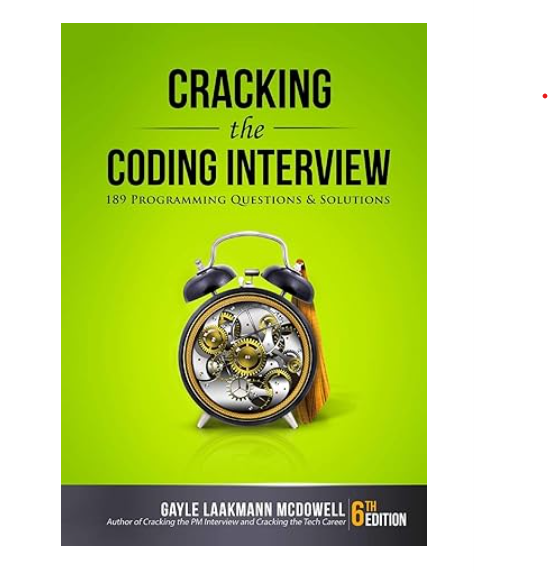
Python ‘pow’ in TypeScript With Example Code
Python’s built-in `pow` function is used to calculate the power of a number. TypeScript, being a superset of JavaScript, does not have a built-in `pow` function. However, we can use the `Math.pow` function in TypeScript to achieve the same result.
To use the `Math.pow` function, we need to pass two arguments: the base number and the exponent. The function returns the result of raising the base number to the power of the exponent.
Here’s an example of how to use the `Math.pow` function in TypeScript:
const base = 2;
const exponent = 3;
const result = Math.pow(base, exponent);
console.log(result); // Output: 8
In this example, we’re calculating 2 raised to the power of 3, which is 8.
We can also use the exponentiation operator (`**`) in TypeScript to achieve the same result. Here’s an example:
const base = 2;
const exponent = 3;
const result = base ** exponent;
console.log(result); // Output: 8
In this example, we’re using the exponentiation operator to calculate 2 raised to the power of 3, which is 8.
In conclusion, while TypeScript does not have a built-in `pow` function, we can use the `Math.pow` function or the exponentiation operator to achieve the same result.
Equivalent of Python pow in TypeScript
In conclusion, the equivalent of the Python pow function in TypeScript is the Math.pow() method. This method takes two arguments, the base and the exponent, and returns the result of raising the base to the power of the exponent. It is a useful tool for performing mathematical calculations in TypeScript, and can be used in a variety of applications. Whether you are working on a simple project or a complex algorithm, the Math.pow() method can help you achieve your goals with ease and efficiency. So, if you are looking for a powerful and reliable way to perform exponentiation in TypeScript, look no further than the Math.pow() method.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |