The range function in Python is used to generate a sequence of numbers. It takes three arguments: start, stop, and step. The start argument is the first number in the sequence, the stop argument is the last number in the sequence (not inclusive), and the step argument is the difference between each number in the sequence. The range function returns a range object, which can be converted to a list or used in a for loop to iterate over the sequence of numbers. The range function is commonly used in Python for generating loops and iterating over a specific range of numbers. Keep reading below to learn how to python range in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
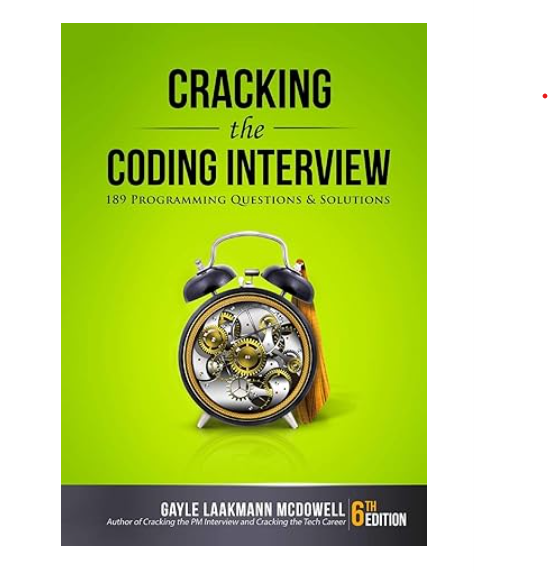
Python ‘range’ in C# With Example Code
Python’s `range()` function is a commonly used tool for generating a sequence of numbers. It is often used in for loops to iterate over a range of values. If you are working in C#, you may be wondering if there is an equivalent function available. While C# does not have a built-in `range()` function, it is possible to create one using a few simple lines of code.
To create a `range()` function in C#, you can use a `yield` statement to generate each value in the sequence. Here is an example implementation:
“` In conclusion, the C# language provides a similar function to Python’s range() function called Enumerable.Range(). This function allows developers to generate a sequence of integers within a specified range, just like the range() function in Python. However, there are some differences between the two functions, such as the fact that Enumerable.Range() returns an IEnumerable object instead of a list. Despite these differences, both functions serve the same purpose and can be used to simplify code and make it more efficient. Whether you’re a Python or C# developer, understanding the range() function and its equivalent in C# can help you write better code and achieve your programming goals more effectively.
public static IEnumerable
{
for (int i = start; i < end; i += step)
{
yield return i;
}
}
```
This function takes three arguments: `start`, `end`, and `step`. `start` is the first value in the sequence, `end` is the last value (which is not included in the sequence), and `step` is the amount to increment by between each value. If `step` is not specified, it defaults to 1.
To use this function, you can call it in a `foreach` loop, like this:
```
foreach (int i in Range(0, 10, 2))
{
Console.WriteLine(i);
}
```
This will output the numbers 0, 2, 4, 6, and 8.
In conclusion, while C# does not have a built-in `range()` function like Python, it is possible to create one using a `yield` statement. This can be useful for generating sequences of numbers in a concise and readable way.
Equivalent of Python range in C#
Elevate your software skills
Ergonomic Mouse
Custom Keyboard
SW Architecture
Clean Code