The range function in Python is used to generate a sequence of numbers. It takes three arguments: start, stop, and step. The start argument is the first number in the sequence, the stop argument is the last number in the sequence (not inclusive), and the step argument is the difference between each number in the sequence. The range function returns a range object, which can be converted to a list or used in a for loop to iterate over the sequence of numbers. The range function is commonly used in Python for generating loops and iterating over a specific range of numbers. Keep reading below to learn how to python range in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
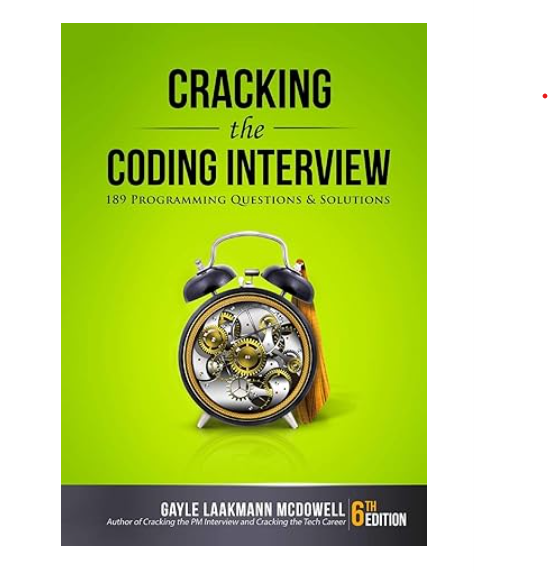
Python ‘range’ in C++ With Example Code
Python’s `range()` function is a commonly used tool for generating a sequence of numbers. It is often used in loops to iterate over a range of values. However, C++ does not have a built-in equivalent to Python’s `range()` function. In this blog post, we will explore how to implement a similar functionality in C++.
One way to implement a `range()` function in C++ is to use a `for` loop. We can define a function that takes in a start value, an end value, and an optional step value. The function can then use a `for` loop to iterate over the range of values and return them in a container such as a vector.
Here is an example implementation of a `range()` function in C++:
“`
#include
std::vector
std::vector
for (int i = start; i < end; i += step) {
result.push_back(i);
}
return result;
}
```
This function takes in a start value, an end value, and an optional step value (which defaults to 1 if not provided). It then creates an empty vector to store the range of values and uses a `for` loop to iterate over the range of values and append them to the vector. Finally, it returns the vector containing the range of values.
We can use this `range()` function in a `for` loop to iterate over a range of values in C++. Here is an example usage:
```
#include
int main() {
for (int i : range(0, 10)) {
std::cout << i << " ";
}
// Output: 0 1 2 3 4 5 6 7 8 9
return 0;
}
```
In this example, we use a `for` loop to iterate over the range of values from 0 to 10 (excluding 10) and print each value to the console.
In conclusion, while C++ does not have a built-in equivalent to Python's `range()` function, we can implement a similar functionality using a `for` loop and a container such as a vector. This allows us to iterate over a range of values in C++ just like we would in Python.
Equivalent of Python range in C++
In conclusion, the equivalent of Python’s range function in C++ is the standard library function “std::iota”. While the syntax and usage may differ slightly, both functions serve the same purpose of generating a sequence of numbers within a given range. It is important to note that the range generated by “std::iota” is inclusive of both the start and end values, unlike Python’s range function which excludes the end value. By understanding the similarities and differences between these two functions, programmers can effectively utilize them in their respective languages to achieve their desired outcomes.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |