The range function in Python is used to generate a sequence of numbers. It takes three arguments: start, stop, and step. The start argument is the first number in the sequence, the stop argument is the last number in the sequence (not inclusive), and the step argument is the difference between each number in the sequence. If the start argument is not specified, it defaults to 0. If the step argument is not specified, it defaults to 1. The range function returns a range object, which can be converted to a list or used in a for loop to iterate over the sequence of numbers. Keep reading below to learn how to python range in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
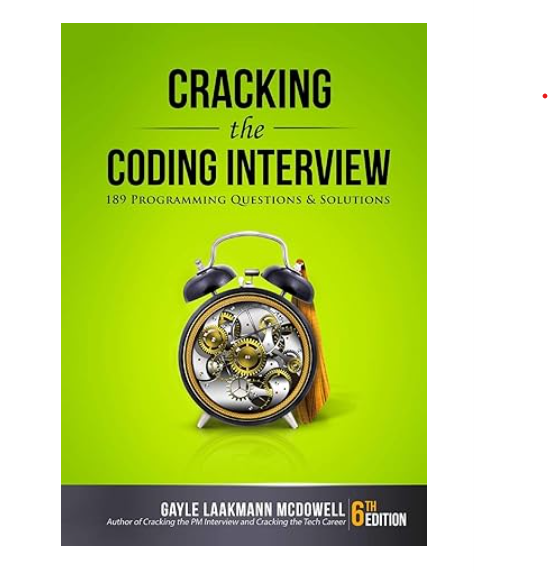
Python ‘range’ in Java With Example Code
Python’s `range()` function is a commonly used tool for generating a sequence of numbers. However, if you are working in Java, you may be wondering how to achieve the same functionality. In this blog post, we will explore how to use Java to replicate the behavior of Python’s `range()` function.
To start, let’s take a look at the syntax of Python’s `range()` function:
“`python
range(start, stop, step)
“`
This function generates a sequence of numbers starting from `start`, up to but not including `stop`, incrementing by `step` each time. If `start` is not specified, it defaults to 0. If `step` is not specified, it defaults to 1.
In Java, we can achieve similar functionality using a `for` loop. Here is an example:
“`java
for (int i = start; i < stop; i += step) {
// Do something with i
}
```
This loop will iterate over a sequence of numbers starting from `start`, up to but not including `stop`, incrementing by `step` each time. If `start` is not specified, it defaults to 0. If `step` is not specified, it defaults to 1.
It's worth noting that Java's `for` loop syntax is slightly different from Python's `range()` function. However, the end result is the same: a sequence of numbers that can be used in a loop.
In conclusion, while Java does not have a built-in `range()` function like Python, we can achieve similar functionality using a `for` loop. By specifying the starting value, ending value, and step size, we can generate a sequence of numbers that can be used in a loop.
Equivalent of Python range in Java
In conclusion, the equivalent of Python’s range function in Java is the for loop. While Python’s range function allows for easy iteration over a range of numbers, Java’s for loop provides similar functionality with a bit more flexibility. By specifying the starting point, ending point, and increment value, Java’s for loop can iterate over a range of numbers just like Python’s range function. Additionally, Java’s for loop can be used to iterate over arrays and collections, making it a versatile tool for any Java programmer. Overall, while the syntax may be different, Java’s for loop provides a powerful and flexible alternative to Python’s range function.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |