The range function in Python is used to generate a sequence of numbers. It takes three arguments: start, stop, and step. The start argument is the first number in the sequence, the stop argument is the last number in the sequence (not inclusive), and the step argument is the difference between each number in the sequence. If the start argument is not specified, it defaults to 0. If the step argument is not specified, it defaults to 1. The range function returns a range object, which can be converted to a list or used in a for loop to iterate over the sequence of numbers. Keep reading below to learn how to python range in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
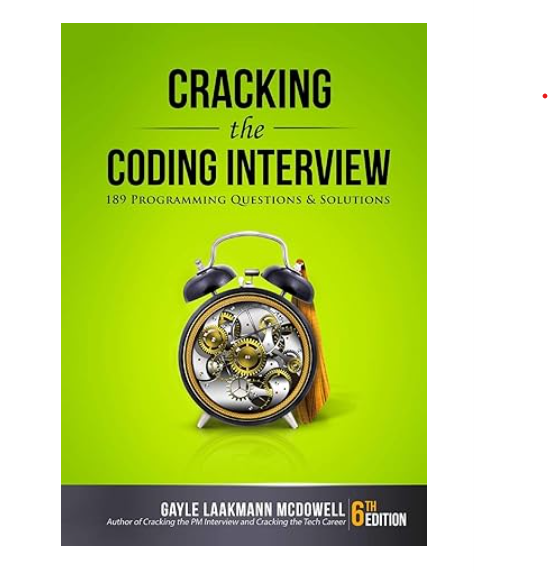
Python ‘range’ in Javascript With Example Code
Python’s `range()` function is a commonly used tool for generating a sequence of numbers. However, if you’re working in JavaScript, you might be wondering how to achieve the same functionality. Fortunately, JavaScript has a similar built-in function called `Array.from()`.
To use `Array.from()` to generate a sequence of numbers, you can pass it an object with a `length` property and a function that returns the desired value for each index. Here’s an example:
const range = (start, stop, step) => Array.from({ length: (stop - start) / step + 1 }, (_, i) => start + (i * step));
In this example, the `range()` function takes three arguments: `start`, `stop`, and `step`. It then uses `Array.from()` to generate an array of numbers starting at `start`, ending at `stop`, and incrementing by `step`.
You can use this `range()` function just like you would use Python’s `range()` function. For example, to generate a sequence of numbers from 0 to 9, you could call `range(0, 9, 1)`.
Keep in mind that `Array.from()` is only available in modern browsers, so if you need to support older browsers, you may need to use a polyfill or a different approach.
Equivalent of Python range in Javascript
In conclusion, the equivalent of Python’s range function in JavaScript is the `Array.from()` method. This method allows us to create an array of a specified length and fill it with values based on a provided function or a simple mapping of indices. It is a powerful tool that can be used in a variety of scenarios, from generating sequences of numbers to creating arrays of objects with specific properties. By understanding the similarities and differences between these two languages, we can leverage the strengths of each to write more efficient and effective code. Whether you’re a seasoned developer or just starting out, knowing how to use the `Array.from()` method in JavaScript can help you write cleaner, more concise code and achieve your programming goals with ease.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |