The range function in Python is used to generate a sequence of numbers. It takes three arguments: start, stop, and step. The start argument is the first number in the sequence, the stop argument is the last number in the sequence (not inclusive), and the step argument is the difference between each number in the sequence. The range function returns a range object, which can be converted to a list or used in a for loop to iterate over the sequence of numbers. The range function is commonly used in Python for generating loops and iterating over a specific range of numbers. Keep reading below to learn how to python range in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
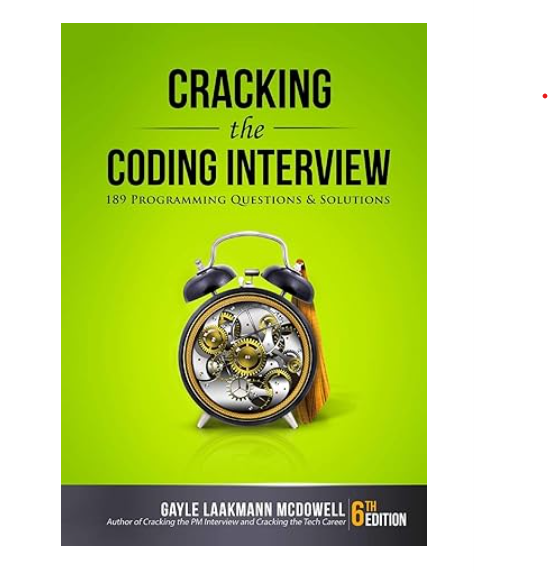
Python ‘range’ in Kotlin With Example Code
Python’s `range()` function is a commonly used tool for generating a sequence of numbers. In Kotlin, there is no direct equivalent to Python’s `range()`, but there are several ways to achieve similar functionality.
One way to generate a sequence of numbers in Kotlin is to use the `IntRange` class. This class represents a range of integers and can be used to iterate over a sequence of numbers. Here’s an example:
val range = 1..10
for (i in range) {
println(i)
}
In this example, we create an `IntRange` object that represents the range of integers from 1 to 10. We then use a `for` loop to iterate over the range and print each number.
Another way to generate a sequence of numbers in Kotlin is to use the `until` function. This function returns a range that does not include the upper bound. Here’s an example:
val range = 1 until 10
for (i in range) {
println(i)
}
In this example, we create a range that includes the integers from 1 to 9. We then use a `for` loop to iterate over the range and print each number.
Finally, you can also use the `step` parameter to generate a sequence of numbers with a specific step size. Here’s an example:
val range = 1..10 step 2
for (i in range) {
println(i)
}
In this example, we create a range that includes the integers from 1 to 10 with a step size of 2. We then use a `for` loop to iterate over the range and print each number.
While Kotlin does not have a direct equivalent to Python’s `range()` function, there are several ways to achieve similar functionality using the `IntRange` class, the `until` function, and the `step` parameter.
Equivalent of Python range in Kotlin
In conclusion, the Kotlin programming language provides a range function that is similar to the range function in Python. The range function in Kotlin is used to create a sequence of numbers that can be iterated over in a loop. It takes two arguments, the start and end values of the range, and an optional step value. The range function in Kotlin is a useful tool for creating loops and iterating over a sequence of numbers. It is a great feature that makes Kotlin a powerful language for developing applications. If you are familiar with the range function in Python, you will find the range function in Kotlin easy to use and understand.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |