The range function in Python is used to generate a sequence of numbers. It takes three arguments: start, stop, and step. The start argument is the first number in the sequence, the stop argument is the last number in the sequence (not inclusive), and the step argument is the difference between each number in the sequence. If the start argument is not specified, it defaults to 0. If the step argument is not specified, it defaults to 1. The range function returns a range object, which can be converted to a list or used in a for loop to iterate over the sequence of numbers. Keep reading below to learn how to python range in Rust.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
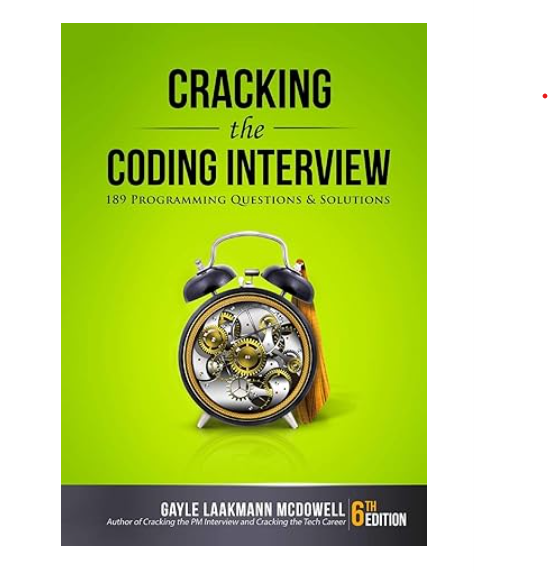
Python ‘range’ in Rust With Example Code
Python’s `range` function is a commonly used tool for generating a sequence of numbers. Rust, being a language with similar syntax and functionality, also has a way to generate ranges of numbers. In this blog post, we will explore how to use Rust’s `Range` type to achieve similar functionality to Python’s `range`.
In Rust, the `Range` type is used to represent a range of values. It is defined as a struct with two fields: `start` and `end`. The `start` field represents the first value in the range, while the `end` field represents the last value in the range. The `Range` type is inclusive of the `start` value and exclusive of the `end` value.
To create a `Range` in Rust, we use the `..` operator. For example, to create a range of numbers from 0 to 9, we can use the following code:
let my_range = 0..10;
This creates a `Range` that includes the values 0 through 9. We can then use this range in a `for` loop to iterate over the values:
for i in my_range {
println!("{}", i);
}
This will print the numbers 0 through 9 to the console.
We can also create a range that starts at a value other than 0. For example, to create a range of numbers from 1 to 10, we can use the following code:
let my_range = 1..11;
This creates a `Range` that includes the values 1 through 10.
In addition to the `..` operator, Rust also provides a `..=` operator to create a range that includes the last value. For example, to create a range of numbers from 1 to 10 inclusive, we can use the following code:
let my_range = 1..=10;
This creates a `Range` that includes the values 1 through 10.
In conclusion, Rust’s `Range` type provides similar functionality to Python’s `range` function. By using the `..` and `..=` operators, we can create ranges of numbers and use them in `for` loops to iterate over the values.
Equivalent of Python range in Rust
In conclusion, the Rust programming language provides a similar functionality to Python’s range function through its built-in iterator types. The range function in Rust can be used to generate a sequence of numbers within a specified range, with the ability to specify the step size and iterate over the sequence using a for loop. Additionally, Rust’s range function can be used with other iterator methods to perform various operations on the generated sequence. Overall, the range function in Rust is a powerful tool for generating and manipulating sequences of numbers, making it a valuable addition to any Rust programmer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |