The round() function in Python is used to round off a given number to a specified number of digits. It takes two arguments, the first being the number to be rounded and the second being the number of digits to round to. If the second argument is not provided, the function rounds the number to the nearest integer. The function uses the standard rounding rules, where numbers ending in 5 are rounded up if the preceding digit is odd and rounded down if the preceding digit is even. The function returns a float if the second argument is provided, otherwise it returns an integer. Keep reading below to learn how to python round in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
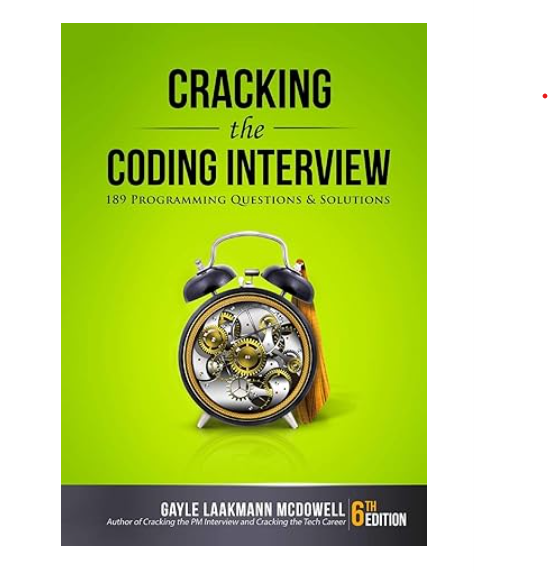
Python ’round’ in C++ With Example Code
Python’s built-in `round()` function is a convenient way to round numbers to a specified number of decimal places. However, if you’re working in C++, you may need to implement rounding functionality yourself. In this blog post, we’ll explore how to do just that.
To start, let’s consider the basic approach to rounding a number in C++. One common method is to add 0.5 to the number and then truncate the result to an integer. Here’s an example implementation:
“`cpp
double round(double num, int decimalPlaces) {
double multiplier = pow(10.0, decimalPlaces);
return floor(num * multiplier + 0.5) / multiplier;
}
“`
In this code, we first calculate a multiplier based on the desired number of decimal places. We then multiply the input number by this multiplier, add 0.5, and take the floor of the result. Finally, we divide by the multiplier to get the rounded result.
Note that this implementation assumes that the input number is positive. If you need to handle negative numbers, you’ll need to adjust the code accordingly.
Another approach to rounding in C++ is to use the `std::round()` function from the `
“`cpp
double round(double num, int decimalPlaces) {
double multiplier = pow(10.0, decimalPlaces);
return std::round(num * multiplier) / multiplier;
}
“`
This code uses the `std::round()` function to round the input number to the nearest integer. We then divide by the multiplier to get the rounded result with the desired number of decimal places.
In conclusion, there are several ways to implement rounding functionality in C++. The approach you choose will depend on your specific requirements and preferences. Whether you use the basic method of adding 0.5 and truncating or the `std::round()` function, you can easily round numbers to a specified number of decimal places in your C++ code.
Equivalent of Python round in C++
In conclusion, the equivalent function to Python’s round() in C++ is the round() function from the
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |