The round() function in Python is used to round off a given number to a specified number of digits. It takes two arguments, the first being the number to be rounded and the second being the number of digits to round to. If the second argument is not provided, the function rounds the number to the nearest integer. The function uses the standard rounding rules, where numbers ending in 5 are rounded up if the preceding digit is odd and rounded down if the preceding digit is even. The function returns a float if the second argument is provided, otherwise it returns an integer. Keep reading below to learn how to python round in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
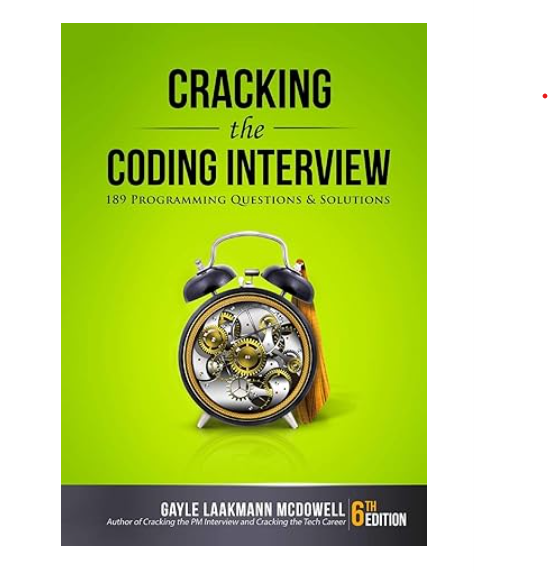
Python ’round’ in Java With Example Code
Python is a popular programming language known for its simplicity and ease of use. However, there may be times when you need to use Java instead. One common task in Python is rounding numbers, and this can also be done in Java. In this blog post, we will show you how to round numbers in Java.
To round a number in Java, you can use the Math.round() method. This method takes a double value as its parameter and returns the closest long value to the argument. Here is an example:
double num = 3.14159;
long roundedNum = Math.round(num);
System.out.println(roundedNum);
In this example, we declare a double variable called num and assign it the value of 3.14159. We then use the Math.round() method to round the number and assign the result to a long variable called roundedNum. Finally, we print out the rounded number using the System.out.println() method.
You can also specify the number of decimal places to round to by using the DecimalFormat class. Here is an example:
double num = 3.14159;
DecimalFormat df = new DecimalFormat("#.##");
double roundedNum = Double.parseDouble(df.format(num));
System.out.println(roundedNum);
In this example, we declare a double variable called num and assign it the value of 3.14159. We then create a DecimalFormat object with the pattern “#.##”, which specifies that we want to round to two decimal places. We use the format() method of the DecimalFormat object to format the number as a string with two decimal places, and then parse the result back into a double using the Double.parseDouble() method. Finally, we print out the rounded number using the System.out.println() method.
In conclusion, rounding numbers in Java is a simple task that can be accomplished using the Math.round() method or the DecimalFormat class. By using these methods, you can easily round numbers to the desired precision in your Java programs.
Equivalent of Python round in Java
In conclusion, the equivalent Java function for the Python round() function is Math.round(). This function takes a floating-point number as input and returns the closest integer value. It works by rounding the number to the nearest whole number, with ties rounding up to the nearest even number. This function is useful for a variety of applications, such as financial calculations, statistical analysis, and scientific research. By understanding the similarities and differences between the Python round() function and the Java Math.round() function, developers can write more efficient and effective code in both languages.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |