The round() function in Python is used to round off a given number to a specified number of digits. It takes two arguments, the first being the number to be rounded and the second being the number of digits to round to. If the second argument is not provided, the function rounds the number to the nearest integer. The function uses the standard rounding rules, where numbers ending in 5 are rounded up if the preceding digit is odd and rounded down if the preceding digit is even. The function returns a float if the second argument is provided, otherwise it returns an integer. Keep reading below to learn how to python round in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
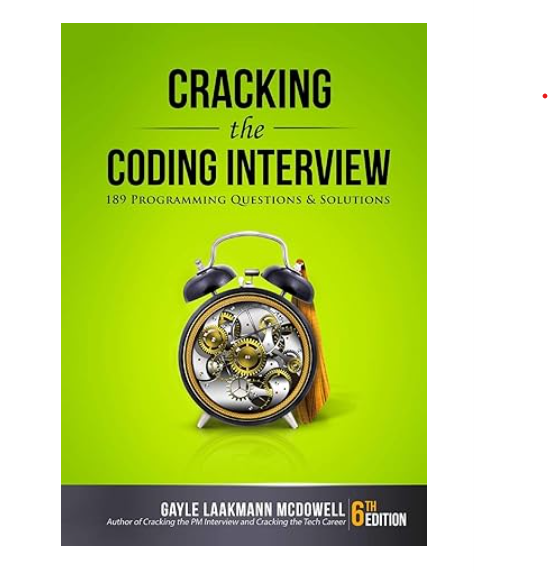
Python ’round’ in Javascript With Example Code
Python’s built-in `round()` function is a useful tool for rounding numbers to a specified number of decimal places. However, if you’re working with JavaScript, you may be wondering how to achieve the same functionality. Fortunately, JavaScript has its own `Math.round()` function that can be used to achieve the same result.
To use `Math.round()` in JavaScript, simply pass the number you want to round as an argument. For example, to round the number `3.14159` to two decimal places, you would use the following code:
Math.round(3.14159 * 100) / 100;
In this example, we first multiply the number by 100 to move the decimal point two places to the right. We then use `Math.round()` to round the number to the nearest integer, and finally divide by 100 to move the decimal point back to its original position.
If you want to round a number to a specific number of decimal places, you can use the following function:
function round(number, precision) {
var factor = Math.pow(10, precision);
return Math.round(number * factor) / factor;
}
This function takes two arguments: the number you want to round, and the number of decimal places you want to round to. It first calculates the factor by which to multiply the number (i.e. 10 to the power of the precision), then uses `Math.round()` to round the number to the nearest integer, and finally divides by the factor to move the decimal point back to its original position.
In conclusion, while Python’s `round()` function may not be available in JavaScript, the `Math.round()` function and the custom `round()` function provided above can be used to achieve the same result.
Equivalent of Python round in Javascript
In conclusion, the equivalent function of Python’s round() in JavaScript is Math.round(). Both functions are used to round off a number to the nearest integer. However, it is important to note that the behavior of the two functions may differ when it comes to rounding off decimal numbers. Python’s round() function has an optional second argument that specifies the number of decimal places to round off to, while JavaScript’s Math.round() function only rounds off to the nearest integer. Therefore, it is important to understand the differences between the two functions and use them appropriately in your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |