In Python, a set is an unordered collection of unique elements. The set() function is used to create a new set object. It takes an iterable object as an argument and returns a set containing all the unique elements from the iterable. If no argument is passed, an empty set is returned. Sets are mutable, meaning that you can add or remove elements from them. Some common operations that can be performed on sets include union, intersection, difference, and symmetric difference. Sets are useful for removing duplicates from a list, checking for membership, and performing mathematical operations on collections of elements. Keep reading below to learn how to python set in Java.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
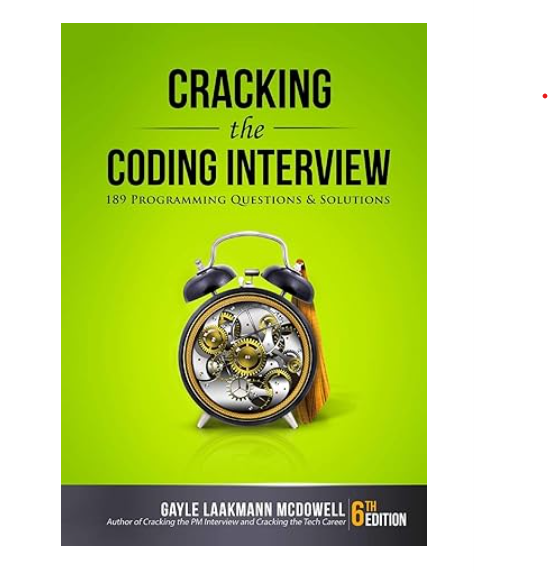
Python ‘set’ in Java With Example Code
Python sets are a powerful data structure that allow for efficient storage and manipulation of unique elements. While Java does not have a built-in set data type, it is possible to implement a set in Java using various approaches.
One approach is to use a HashSet, which is a class in the Java Collections framework that implements the Set interface. A HashSet stores elements in a hash table, which allows for constant-time performance for basic operations such as add, remove, and contains.
To create a HashSet in Java, you can use the following code:
“`
Set
“`
This creates a new HashSet that stores elements of type String. You can then add elements to the set using the add() method:
“`
mySet.add(“apple”);
mySet.add(“banana”);
mySet.add(“orange”);
“`
To check if an element is in the set, you can use the contains() method:
“`
if (mySet.contains(“apple”)) {
System.out.println(“The set contains apple”);
}
“`
To remove an element from the set, you can use the remove() method:
“`
mySet.remove(“banana”);
“`
You can also iterate over the elements in the set using a for-each loop:
“`
for (String element : mySet) {
System.out.println(element);
}
“`
This will print out each element in the set in an arbitrary order.
In summary, while Java does not have a built-in set data type, it is possible to implement a set using a HashSet. This allows for efficient storage and manipulation of unique elements in Java.
Equivalent of Python set in Java
In conclusion, the equivalent Java function for Python’s set() is the HashSet class. Both set() and HashSet allow for the creation of a collection of unique elements, with the ability to perform set operations such as union, intersection, and difference. While the syntax and implementation may differ between the two languages, the fundamental concept of a set remains the same. As a Java developer, understanding the HashSet class and its methods can greatly enhance your ability to work with collections of unique elements in your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |