In Python, a set is an unordered collection of unique elements. The set() function is used to create a new set object. It takes an iterable object as an argument and returns a set containing all the unique elements from the iterable. If no argument is passed, an empty set is returned. Sets are mutable, meaning that you can add or remove elements from them. Some common operations that can be performed on sets include union, intersection, difference, and symmetric difference. Sets are useful for removing duplicates from a list, checking for membership, and performing mathematical operations on collections of elements. Keep reading below to learn how to python set in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
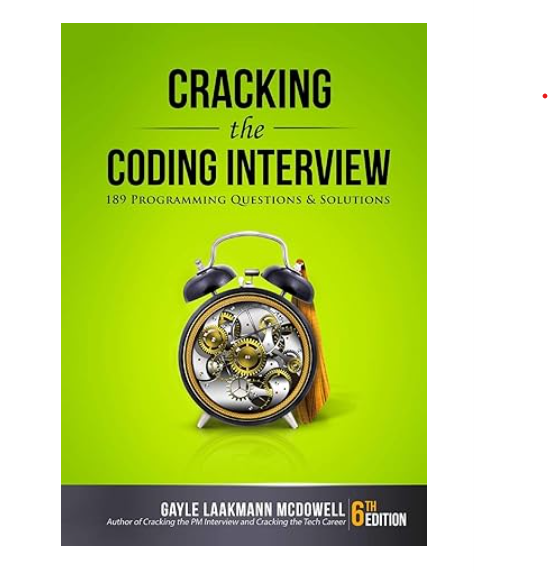
Python ‘set’ in Kotlin With Example Code
Python sets are a powerful data structure that allow for efficient storage and manipulation of unique elements. Kotlin, being a modern and versatile programming language, also provides a similar data structure for developers to use. In this blog post, we will explore how to use Python sets in Kotlin.
To create a set in Kotlin, we can use the `setOf()` function. This function takes in a variable number of arguments and returns a set containing those elements. For example, to create a set containing the numbers 1, 2, and 3, we can use the following code:
val mySet = setOf(1, 2, 3)
We can also create an empty set using the `emptySet()` function. This function returns a read-only empty set that can be used as a starting point for adding elements later on. For example:
val myEmptySet = emptySet<Int>()
To add elements to a set, we can use the `plus()` function. This function takes in an element and returns a new set with that element added. For example:
val mySet = setOf(1, 2, 3)
val newSet = mySet.plus(4)
In this example, `newSet` will contain the elements 1, 2, 3, and 4.
To remove elements from a set, we can use the `minus()` function. This function takes in an element and returns a new set with that element removed. For example:
val mySet = setOf(1, 2, 3)
val newSet = mySet.minus(2)
In this example, `newSet` will contain the elements 1 and 3.
We can also perform set operations such as union, intersection, and difference using the appropriate functions. For example:
val set1 = setOf(1, 2, 3)
val set2 = setOf(3, 4, 5)
val unionSet = set1.union(set2)
val intersectionSet = set1.intersect(set2)
val differenceSet = set1.subtract(set2)
In this example, `unionSet` will contain the elements 1, 2, 3, 4, and 5, `intersectionSet` will contain the element 3, and `differenceSet` will contain the elements 1 and 2.
In conclusion, Kotlin provides a powerful set data structure that is similar to Python sets. By using the functions provided by Kotlin, we can efficiently store and manipulate unique elements in our programs.
Equivalent of Python set in Kotlin
In conclusion, Kotlin provides a powerful set of functions that make it easy to work with sets of data. One of these functions is the equivalent of the Python set() function, which allows you to create a set from a list or other iterable object. This function is called toSet() in Kotlin and can be used to quickly and easily create sets that can be manipulated and analyzed in a variety of ways. Whether you are working with large datasets or just need to perform simple set operations, Kotlin’s set functions provide a flexible and efficient way to get the job done. So, if you are looking for a modern programming language that offers robust set functionality, Kotlin is definitely worth considering.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |