The str() function in Python is used to convert any data type into a string. It takes an object as an argument and returns a string representation of that object. This function is particularly useful when we need to concatenate strings with other data types like integers or floats. It can also be used to format strings by inserting variables into a string using placeholders. The str() function is a built-in function in Python, which means it is available for use without the need for any additional libraries or modules.. Keep reading below to learn how to python str in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
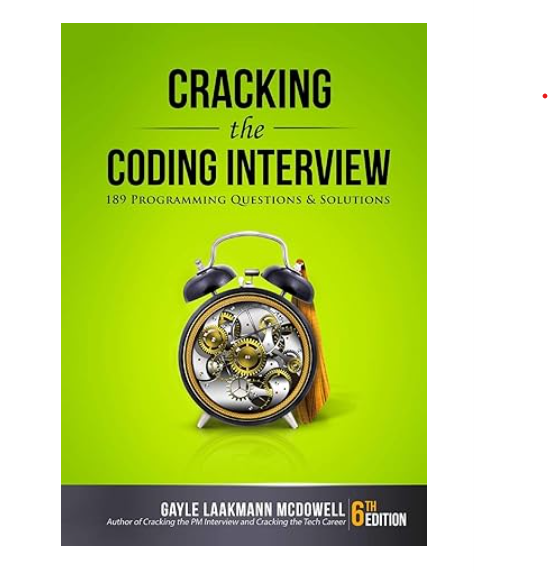
Python ‘str’ in C# With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. One of the most commonly used data types in Python is the string. In this blog post, we will explore how to work with Python strings in C#.
To begin, let’s first understand what a string is. A string is a sequence of characters that is used to represent text. In Python, strings are represented using single quotes (‘ ‘) or double quotes (” “). For example, the following code creates a string in Python:
“`
my_string = “Hello, World!”
“`
Now, let’s see how we can work with Python strings in C#. C# provides a class called `System.String` that is used to represent strings. To create a string in C#, we can simply use double quotes (” “) or use the `string` keyword. For example:
“`
string myString = “Hello, World!”;
“`
To access individual characters in a string, we can use the indexing operator ([]). For example, to access the first character in a string, we can use the following code:
“`
char firstChar = myString[0];
“`
C# also provides a number of methods that can be used to manipulate strings. For example, the `ToUpper()` method can be used to convert a string to uppercase:
“`
string myString = “Hello, World!”;
string upperCaseString = myString.ToUpper();
“`
Similarly, the `ToLower()` method can be used to convert a string to lowercase:
“`
string myString = “Hello, World!”;
string lowerCaseString = myString.ToLower();
“`
In addition to these methods, C# also provides a number of other methods that can be used to manipulate strings, such as `Substring()`, `Replace()`, and `Split()`.
In conclusion, working with Python strings in C# is fairly straightforward. C# provides a number of methods that can be used to manipulate strings, and the `System.String` class can be used to represent strings in C#.
Equivalent of Python str in C#
In conclusion, the equivalent function to Python’s str() in C# is the ToString() method. Both functions serve the same purpose of converting a value to a string representation. However, the syntax and usage of the two functions differ slightly. While Python’s str() function is a built-in function that can be called directly on a value, C#’s ToString() method is a method that must be called on an object. Despite these differences, both functions are essential in converting values to strings, which is a crucial aspect of programming. As a programmer, it is important to understand the similarities and differences between these functions to effectively use them in your code.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |