The Python sum() function is a built-in function that takes an iterable (such as a list, tuple, or set) as its argument and returns the sum of all the elements in the iterable. It can also take an optional second argument, which is the starting value for the sum. If the iterable contains non-numeric elements, the sum() function will raise a TypeError. The sum() function is a convenient way to quickly calculate the total of a list of numbers or other iterable objects.. Keep reading below to learn how to python sum in C++.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
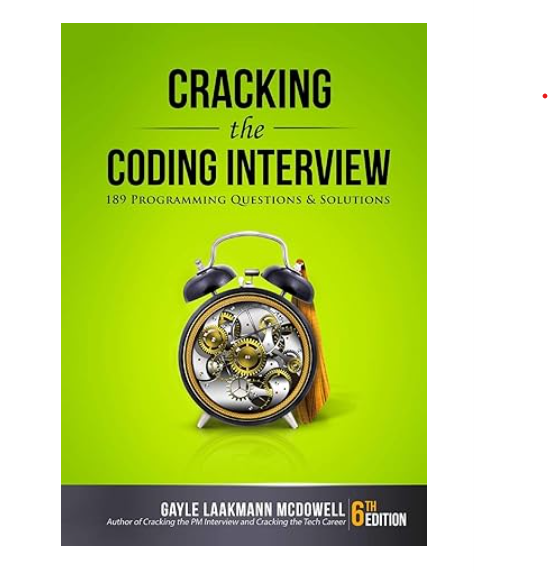
Python ‘sum’ in C++ With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. One of the most commonly used functions in Python is the sum() function, which is used to add up a list of numbers. If you are a C++ programmer, you may be wondering how to achieve the same functionality in C++. In this blog post, we will show you how to implement the Python sum() function in C++.
The first step in implementing the sum() function in C++ is to create a function that takes in a list of numbers as a parameter. In C++, this can be done using an array or a vector. For the purpose of this tutorial, we will be using a vector.
Here is the code for the function:
double sum(std::vector<double> numbers) {
double total = 0;
for (int i = 0; i < numbers.size(); i++) {
total += numbers[i];
}
return total;
}
As you can see, the function takes in a vector of doubles and returns the sum of all the numbers in the vector. The function uses a for loop to iterate through the vector and add up all the numbers.
Now that we have our sum() function, we can use it to add up a list of numbers in C++. Here is an example:
std::vector<double> numbers = {1.0, 2.0, 3.0, 4.0, 5.0};
double total = sum(numbers);
std::cout << total << std::endl;
In this example, we create a vector of doubles containing the numbers 1.0 through 5.0. We then call our sum() function and pass in the vector as a parameter. The function returns the sum of all the numbers in the vector, which we store in the variable total. Finally, we print out the value of total using the cout statement.
And that’s it! You now know how to implement the Python sum() function in C++. Happy coding!
Equivalent of Python sum in C++
In conclusion, the equivalent of the Python sum function in C++ is the accumulate function. Both functions are used to calculate the sum of elements in a container. However, the accumulate function in C++ requires the use of iterators and an initial value for the sum. Despite this difference, the accumulate function is a powerful tool in C++ for performing mathematical operations on containers. By understanding the similarities and differences between these two functions, programmers can choose the best tool for their specific needs. Overall, the accumulate function is a valuable addition to any C++ programmer’s toolkit.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |