In Python, a tuple is an ordered collection of elements, similar to a list. However, unlike lists, tuples are immutable, meaning that their contents cannot be changed once they are created. Tuples are defined using parentheses, with each element separated by a comma. They can contain any type of data, including other tuples. Tuples are often used to group related data together, and can be accessed using indexing or slicing. They are also commonly used as function arguments and return values, as they provide a convenient way to pass multiple values around without having to create a separate data structure.. Keep reading below to learn how to python tuple in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
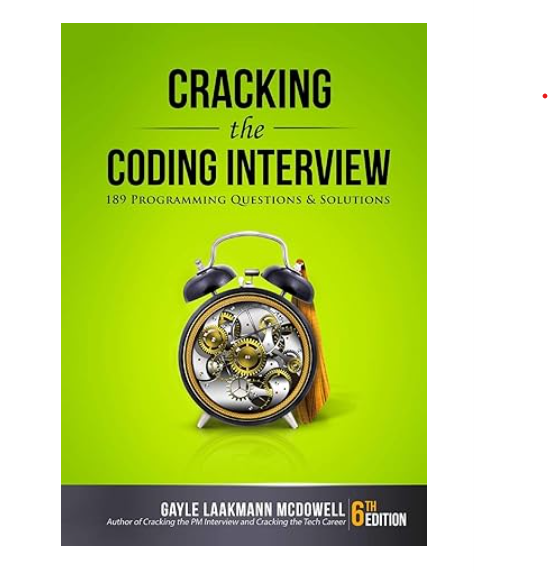
Python ‘tuple’ in C# With Example Code
Python is a popular programming language that is known for its simplicity and ease of use. One of the data structures that Python uses is the tuple. A tuple is an ordered collection of elements that can be of different data types. In Python, tuples are immutable, which means that once they are created, their values cannot be changed.
If you are a C# developer, you may be wondering how to use tuples in C#. Fortunately, C# 7 introduced support for tuples, making it easy to work with them in your code.
To create a tuple in C#, you can use the Tuple class. Here is an example:
Tuple<int, string> tuple = new Tuple<int, string>(1, "hello");
This creates a tuple with two elements: an integer with a value of 1, and a string with a value of “hello”.
You can also use the new tuple syntax introduced in C# 7. Here is an example:
(int, string) tuple = (1, "hello");
This creates a tuple with the same values as the previous example, but using the new syntax.
To access the elements of a tuple in C#, you can use the Item property of the Tuple class, or you can use deconstruction. Here is an example:
int first = tuple.Item1;
string second = tuple.Item2;
This code accesses the elements of the tuple using the Item property.
Alternatively, you can use deconstruction to assign the values of the tuple to separate variables:
(int first, string second) = tuple;
This code assigns the values of the tuple to the variables first and second using deconstruction.
In conclusion, tuples are a useful data structure in Python, and C# 7 introduced support for tuples, making it easy to work with them in your C# code.
Equivalent of Python tuple in C#
In conclusion, the equivalent function of Python’s tuple in C# is the Tuple class. This class provides a convenient way to group multiple values into a single object, just like a tuple in Python. The Tuple class is available in C# 4.0 and later versions and can be used to create tuples of up to eight elements. It is a useful tool for developers who need to work with multiple values and want to keep them organized and easily accessible. With the Tuple class, C# developers can enjoy the same benefits of tuples as Python developers, making their code more efficient and readable.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |