In Python, a tuple is an ordered collection of elements, similar to a list. However, unlike lists, tuples are immutable, meaning that their contents cannot be changed once they are created. Tuples are defined using parentheses, with each element separated by a comma. They can contain any type of data, including other tuples. Tuples are often used to group related data together, and can be accessed using indexing or slicing. They are also commonly used as function arguments and return values, as they provide a convenient way to pass multiple values around without having to create a separate data structure. Keep reading below to learn how to python tuple in Javascript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
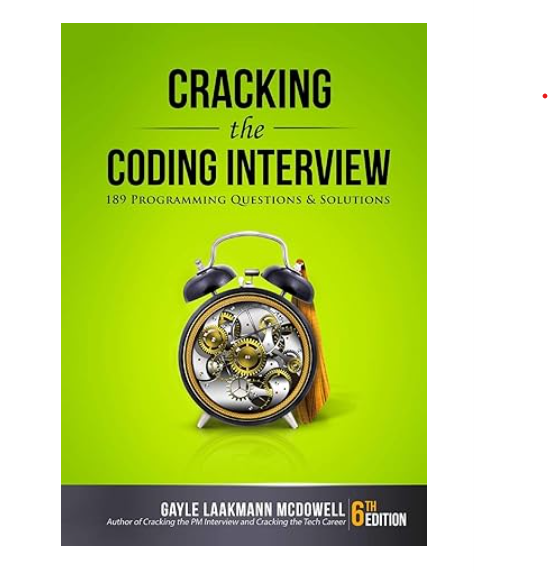
Python ‘tuple’ in Javascript With Example Code
Python tuples are a convenient way to store multiple values in a single variable. They are immutable, meaning that once a tuple is created, its values cannot be changed. In JavaScript, there is no built-in tuple data type, but we can use arrays to achieve similar functionality.
To create a tuple-like structure in JavaScript, we can simply create an array with the desired values:
const myTuple = ['apple', 'banana', 'cherry'];
We can access individual values in the tuple using array indexing:
console.log(myTuple[0]); // Output: 'apple'
Just like with Python tuples, we cannot modify the values in the array:
myTuple[0] = 'orange'; // This will throw an error
However, we can still perform operations on the tuple, such as concatenation:
const myOtherTuple = ['date', 'elderberry'];
const concatenatedTuple = myTuple.concat(myOtherTuple);
Now, concatenatedTuple
contains all the values from both tuples:
console.log(concatenatedTuple); // Output: ['apple', 'banana', 'cherry', 'date', 'elderberry']
Overall, while JavaScript does not have a built-in tuple data type, we can use arrays to achieve similar functionality.
Equivalent of Python tuple in Javascript
In conclusion, while Python and JavaScript are two different programming languages, they share some similarities in their syntax and functionality. One such similarity is the concept of tuples, which are immutable ordered collections of elements. While Python has a built-in tuple function, JavaScript does not have an equivalent function. However, there are several ways to create tuples in JavaScript, such as using arrays or objects. By understanding the differences between tuples in Python and JavaScript, developers can effectively use these data structures in their code to improve performance and readability. Overall, while the lack of a built-in tuple function in JavaScript may seem like a limitation, it is important to remember that there are always alternative solutions available.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |