In Python, a tuple is an ordered collection of elements, similar to a list. However, unlike lists, tuples are immutable, meaning that their contents cannot be changed once they are created. Tuples are defined using parentheses, with each element separated by a comma. They can contain any type of data, including other tuples. Tuples are often used to group related data together, and can be accessed using indexing or slicing. They are also commonly used as function arguments and return values, as they provide a convenient way to pass multiple values around without having to create a separate data structure. Keep reading below to learn how to python tuple in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
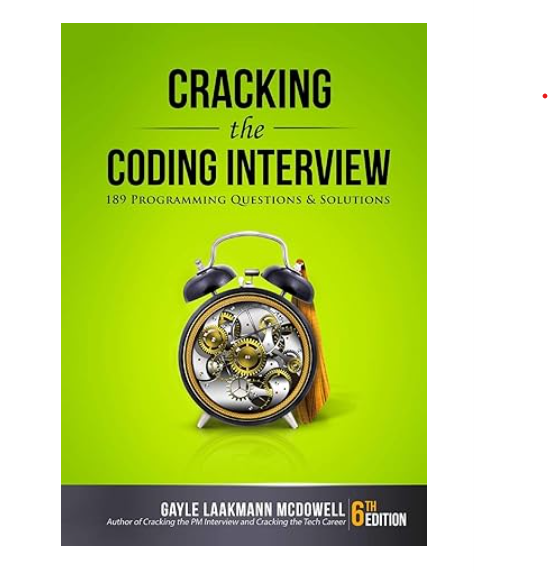
Python ‘tuple’ in Kotlin With Example Code
Python is a popular programming language that is widely used for various purposes. One of its features is the tuple data type, which is an ordered, immutable collection of elements. Kotlin, another popular programming language, also has a similar data type called the Pair. In this blog post, we will explore how to use Python tuples in Kotlin.
To use Python tuples in Kotlin, we can create a Pair object with the elements of the tuple. For example, if we have a Python tuple with two elements, we can create a Pair object in Kotlin like this:
val myTuple = (1, "hello")
val myPair = Pair(myTuple[0], myTuple[1])
In this example, we first create a Python tuple with two elements, 1 and “hello”. We then create a Pair object in Kotlin with the first element of the tuple as the first element of the Pair, and the second element of the tuple as the second element of the Pair.
We can also create a Pair object with a Python tuple using destructuring declarations. For example:
val (x, y) = (1, "hello")
val myPair = Pair(x, y)
In this example, we use destructuring declarations to assign the elements of the Python tuple to variables x and y. We then create a Pair object with these variables.
In conclusion, using Python tuples in Kotlin is easy with the Pair data type. We can create a Pair object with the elements of the tuple, or use destructuring declarations to assign the elements of the tuple to variables and then create a Pair object.
Equivalent of Python tuple in Kotlin
In conclusion, Kotlin provides a convenient and efficient way to work with tuples through its built-in Pair and Triple classes. These classes allow developers to group multiple values together and pass them around as a single object, similar to Python’s tuple function. Additionally, Kotlin’s type inference and concise syntax make it easy to create and manipulate tuples in a clean and readable way. Whether you’re working on a small project or a large-scale application, Kotlin’s tuple functionality can help simplify your code and improve its overall readability and maintainability.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |