In Python, a tuple is an ordered collection of elements, similar to a list. However, unlike lists, tuples are immutable, meaning that their contents cannot be changed once they are created. Tuples are defined using parentheses, with each element separated by a comma. They can contain any type of data, including other tuples. Tuples are often used to group related data together, and can be accessed using indexing or slicing. They are also commonly used as function arguments and return values, as they provide a convenient way to pass multiple values around without having to create a separate data structure. Keep reading below to learn how to python tuple in TypeScript.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
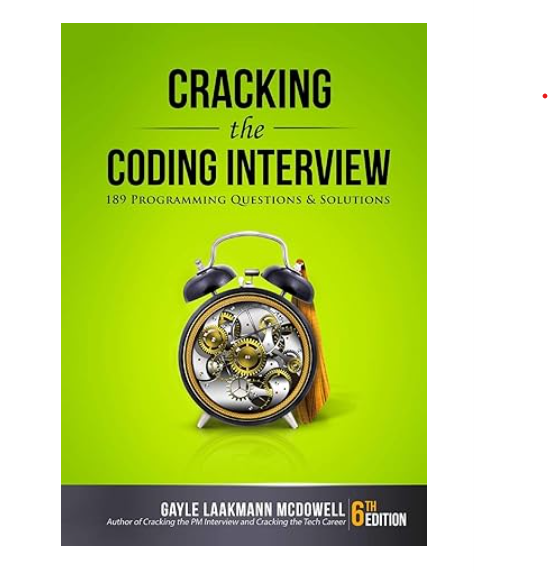
Python ‘tuple’ in TypeScript With Example Code
Python tuples are a convenient way to store multiple values in a single variable. In TypeScript, tuples can also be used to achieve the same functionality. In this blog post, we will explore how to use Python tuples in TypeScript.
To create a tuple in TypeScript, we can use the following syntax:
let myTuple: [string, number] = ["hello", 10];
This creates a tuple with two elements – a string and a number. We can access the elements of the tuple using their index:
console.log(myTuple[0]); // Output: "hello"
console.log(myTuple[1]); // Output: 10
We can also use destructuring to assign the elements of the tuple to separate variables:
let [myString, myNumber] = myTuple;
console.log(myString); // Output: "hello"
console.log(myNumber); // Output: 10
Tuples can also be used as function parameters and return types:
function myFunction(myTuple: [string, number]): void {
console.log(myTuple[0]);
console.log(myTuple[1]);
}
myFunction(["hello", 10]); // Output: "hello" and 10
In conclusion, Python tuples can be easily used in TypeScript to store multiple values in a single variable. With the ability to access tuple elements using their index and use them as function parameters and return types, tuples can be a powerful tool in TypeScript development.
Equivalent of Python tuple in TypeScript
In conclusion, TypeScript provides a similar function to Python’s tuple called “readonly tuple”. This function allows developers to create an immutable array of values with a fixed length. The “readonly” keyword ensures that the tuple cannot be modified once it is created, providing a level of type safety and preventing accidental changes to the data. Using tuples in TypeScript can be particularly useful when working with APIs that return fixed-length arrays or when defining function parameters that require a specific number and type of arguments. Additionally, tuples can be used in conjunction with other TypeScript features such as union types and destructuring, making them a powerful tool in any TypeScript developer’s toolkit.Overall, the “readonly tuple” function in TypeScript provides a convenient and safe way to work with fixed-length arrays of data, and is a valuable addition to the language’s feature set.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |