The Python zip function is a built-in function that takes two or more iterables as arguments and returns an iterator that aggregates the elements from each of the iterables. The resulting iterator contains tuples where the i-th tuple contains the i-th element from each of the input iterables. If the input iterables are of different lengths, the resulting iterator will have a length equal to the shortest input iterable. The zip function is commonly used to combine two or more lists or tuples into a single iterable for processing.. Keep reading below to learn how to python zip in C#.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
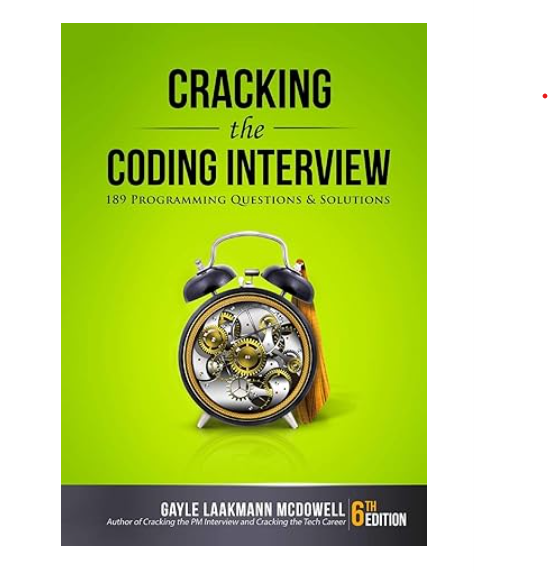
Python ‘zip’ in C# With Example Code
Python’s `zip` function is a powerful tool for combining multiple lists into a single list of tuples. If you’re working in C#, you might be wondering how to achieve the same functionality. Fortunately, C# provides a similar method for zipping lists together.
To zip two lists together in C#, you can use the `Zip` method provided by the `System.Linq` namespace. This method takes two lists as arguments and returns a new list of tuples, where each tuple contains one element from each of the input lists.
Here’s an example of how to use the `Zip` method:
“`csharp
using System;
using System.Linq;
class Program
{
static void Main()
{
var list1 = new[] { 1, 2, 3 };
var list2 = new[] { “one”, “two”, “three” };
var zipped = list1.Zip(list2, (i, s) => Tuple.Create(i, s));
foreach (var tuple in zipped)
{
Console.WriteLine(“{0} – {1}”, tuple.Item1, tuple.Item2);
}
}
}
“`
In this example, we have two lists: `list1` contains integers, and `list2` contains strings. We use the `Zip` method to combine these lists into a new list of tuples, where each tuple contains one integer and one string. We then iterate over the tuples and print out each element.
Note that the `Zip` method takes a third argument, which is a function that specifies how to combine the elements from the input lists into a single tuple. In this example, we use the `Tuple.Create` method to create a new tuple with one integer and one string.
In conclusion, while C# doesn’t have a built-in `zip` function like Python, the `Zip` method provided by the `System.Linq` namespace provides similar functionality. By using this method, you can easily combine multiple lists into a single list of tuples.
Equivalent of Python zip in C#
In conclusion, the equivalent of the Python zip function in C# is the Enumerable.Zip method. This method allows developers to combine multiple sequences into a single sequence of tuples, just like the Python zip function. The Zip method is a powerful tool for data manipulation and can be used in a variety of scenarios, such as merging data from different sources or creating new data structures. With the Zip method, C# developers can enjoy the same functionality as Python developers when it comes to combining sequences. Overall, the Zip method is a valuable addition to the C# language and is worth exploring for any developer looking to streamline their data manipulation processes.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |