The Python zip function is a built-in function that takes two or more iterables as arguments and returns an iterator that aggregates the elements from each of the iterables. The resulting iterator contains tuples where the i-th tuple contains the i-th element from each of the input iterables. If the input iterables are of different lengths, the resulting iterator will have a length equal to the shortest input iterable. The zip function is commonly used to combine two or more lists or tuples into a single iterable for processing. Keep reading below to learn how to python zip in Go.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
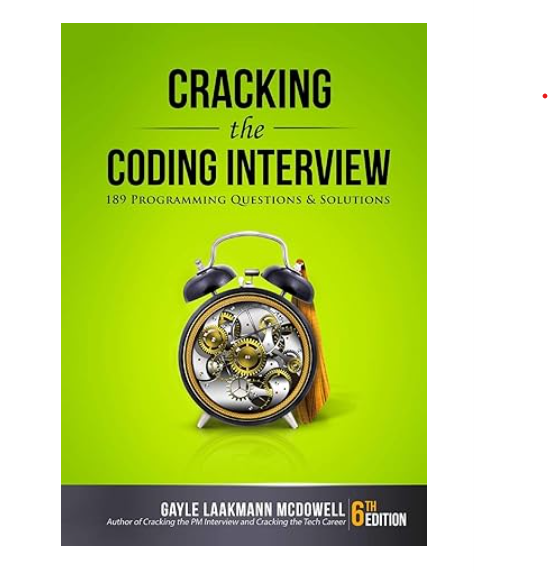
Python ‘zip’ in Go With Example Code
Python’s `zip` function is a powerful tool for combining multiple lists into a single list of tuples. But what if you’re working in Go and need to achieve the same result? Fortunately, Go provides a similar function called `zip`.
To use `zip` in Go, you’ll need to import the `github.com/ericlagergren/iter` package. This package provides a variety of functions for working with iterators, including `Zip`.
Here’s an example of how to use `Zip` to combine two slices of integers into a single slice of tuples:
package main
import (
"fmt"
"github.com/ericlagergren/iter"
)
func main() {
a := []int{1, 2, 3}
b := []int{4, 5, 6}
zipped := iter.Zip(iter.Ints(a), iter.Ints(b))
for _, tuple := range zipped {
fmt.Println(tuple)
}
}
In this example, we create two slices of integers (`a` and `b`) and then use `Zip` to combine them into a single slice of tuples (`zipped`). We then loop over the tuples and print them out.
Note that `Zip` returns an iterator, not a slice. If you need a slice, you can convert the iterator to a slice using the `iter.ToSlice` function:
zippedSlice := iter.ToSlice(zipped).([][2]int)
This will give you a slice of `[2]int` tuples.
With `Zip`, you can easily combine multiple slices into a single slice of tuples in Go, just like you would with Python’s `zip` function.
Equivalent of Python zip in Go
In conclusion, the equivalent of Python’s zip function in Go is the `zip` package. This package provides a simple and efficient way to combine multiple slices into a single slice of tuples. The `zip` function takes in any number of slices of the same type and returns a slice of tuples, where each tuple contains the corresponding elements from each input slice. This functionality is particularly useful when working with large datasets or when performing complex data manipulations. With the `zip` package, Go developers can easily perform operations that would otherwise require multiple loops or complex logic. Overall, the `zip` package is a valuable addition to the Go language and a powerful tool for any developer working with slices.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |