The Python zip function is a built-in function that takes two or more iterables as arguments and returns an iterator that aggregates the elements from each of the iterables. The resulting iterator contains tuples where the i-th tuple contains the i-th element from each of the input iterables. If the input iterables are of different lengths, the resulting iterator will have a length equal to the shortest input iterable. The zip function is commonly used to combine two or more lists or tuples into a single iterable for processing. Keep reading below to learn how to python zip in Kotlin.
Looking to get a head start on your next software interview? Pickup a copy of the best book to prepare: Cracking The Coding Interview!
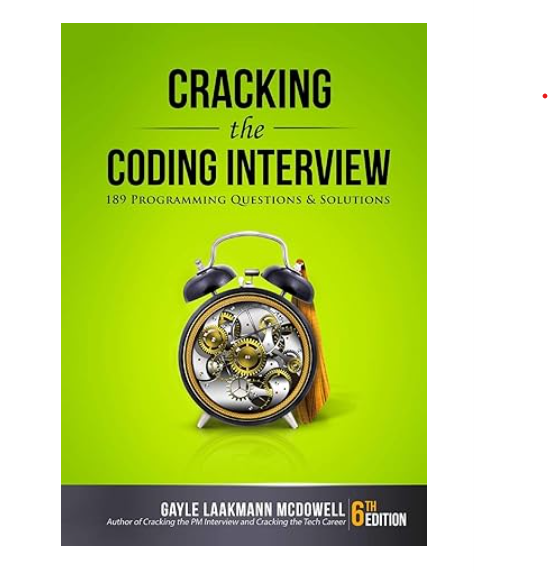
Python ‘zip’ in Kotlin With Example Code
Python’s `zip` function is a useful tool for combining multiple lists into a single list of tuples. Kotlin, being a language that is heavily influenced by Python, also has a similar function called `zip`. In this blog post, we will explore how to use `zip` in Kotlin.
To use `zip` in Kotlin, you simply call the `zip` function on two or more lists and it will return a list of tuples containing the corresponding elements from each list. For example:
val list1 = listOf("a", "b", "c")
val list2 = listOf(1, 2, 3)
val zipped = list1.zip(list2)
println(zipped) // [(a, 1), (b, 2), (c, 3)]
In the example above, we have two lists `list1` and `list2` containing strings and integers respectively. We then call the `zip` function on `list1` and pass `list2` as an argument. The resulting list `zipped` contains tuples of corresponding elements from both lists.
You can also use `zip` with more than two lists. For example:
val list1 = listOf("a", "b", "c")
val list2 = listOf(1, 2, 3)
val list3 = listOf(true, false, true)
val zipped = list1.zip(list2, list3)
println(zipped) // [(a, 1, true), (b, 2, false), (c, 3, true)]
In the example above, we have three lists `list1`, `list2`, and `list3`. We then call the `zip` function on `list1` and pass `list2` and `list3` as arguments. The resulting list `zipped` contains tuples of corresponding elements from all three lists.
In conclusion, `zip` is a useful function in Kotlin for combining multiple lists into a single list of tuples. It is easy to use and can be used with any number of lists.
Equivalent of Python zip in Kotlin
In conclusion, the Kotlin programming language provides a convenient and efficient way to work with collections through its zip function. This function allows developers to combine two or more collections into a single collection of pairs, which can be useful in a variety of scenarios. The zip function in Kotlin is similar to the zip function in Python, but with some differences in syntax and behavior. By using the zip function in Kotlin, developers can simplify their code and improve its readability, making it easier to maintain and debug. Overall, the zip function in Kotlin is a powerful tool that can help developers work with collections more effectively and efficiently.
Elevate your software skills
Ergonomic Mouse![]() |
Custom Keyboard![]() |
SW Architecture![]() |
Clean Code![]() |